API
This guide is targeted to developers who want to access Autonomous Identity using the REST Application Programming Interface (API).
ForgeRock® Autonomous Identity is an entitlements analytics system that lets you fully manage your company’s access to your data.
An entitlement refers to the rights or privileges assigned to a user or thing for access to specific resources. A company can have millions of entitlements without a clear picture of what they are, what they do, and who they are assigned to. Autonomous Identity solves this problem by using advanced artificial intelligence (AI) and automation technology to determine the full entitlements landscape for your company. The system also detects potential risks arising from incorrect or over-provisioned entitlements that lead to policy violations. Autonomous Identity eliminates the manual re-certification of entitlements and provides a centralized, transparent, and contextual view of all access points within your company.
About the Autonomous Identity API
Autonomous Identity provides a RESTful application programming interface (API) that lets you use HTTP request methods (GET, PUT, and POST) to interact with the system and its components. The API lets a developer make requests to send or receive data to an Autonomous Identity endpoint, a point where the API communicates with the system. The data that is sent or returned is in JavaScript Object Notation (JSON) format.
Autonomous Identity provides a Swagger client that you can access on the console.
Swagger
The Autonomous Identity installs with a Swagger client that lets you interact with the Autonomous Identity API and the configuration service API. Swagger is a popular software that provides design, build, test, and documentation tools for RESTful APIs.
-
Open a browser, and point it to
https://autoid-ui.forgerock.com/
. Log in to the Autonomous Identity console. -
Open a browser, and point it to
https://autoid-api.forgerock.com/endpoints/
.
See it in action
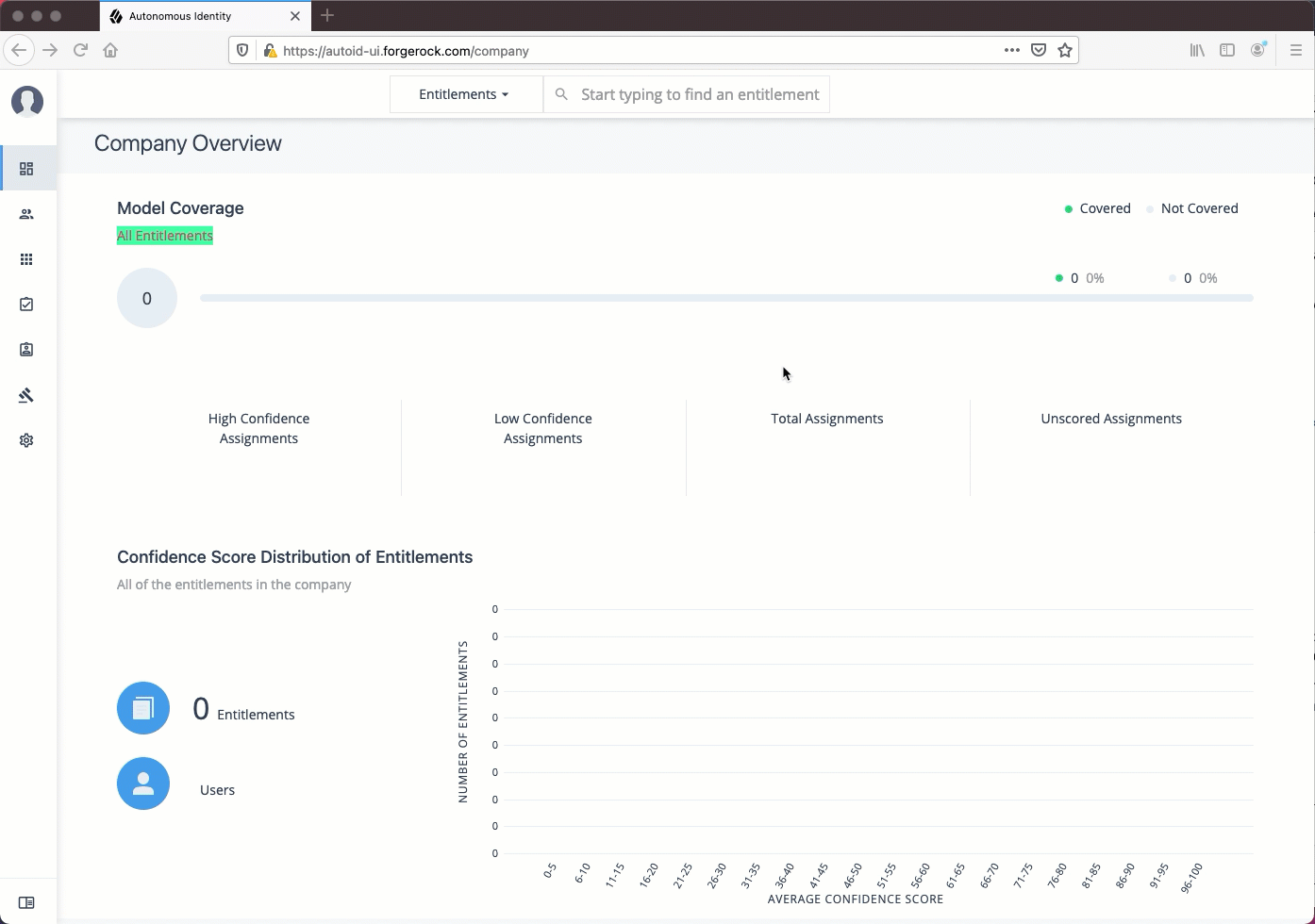
-
Open a browser, and point it to
https://autoid-ui.forgerock.com/
. Log in to the Autonomous Identity console. -
Open another browser tab, and point to
https://autoid-ui.forgerock.com/swagger/
. You should see a default Swagger API page. -
Open another browser tab, and point to
https://autoid-ui.forgerock.com/api/swagger
. You should see a raw text version of the API. -
Go back to the Swagger page in step 2, and enter
https://autoid-ui.forgerock.com/api/swagger
in the field, and click Explore. You will see the Autonomous Identity API service.
See it in action
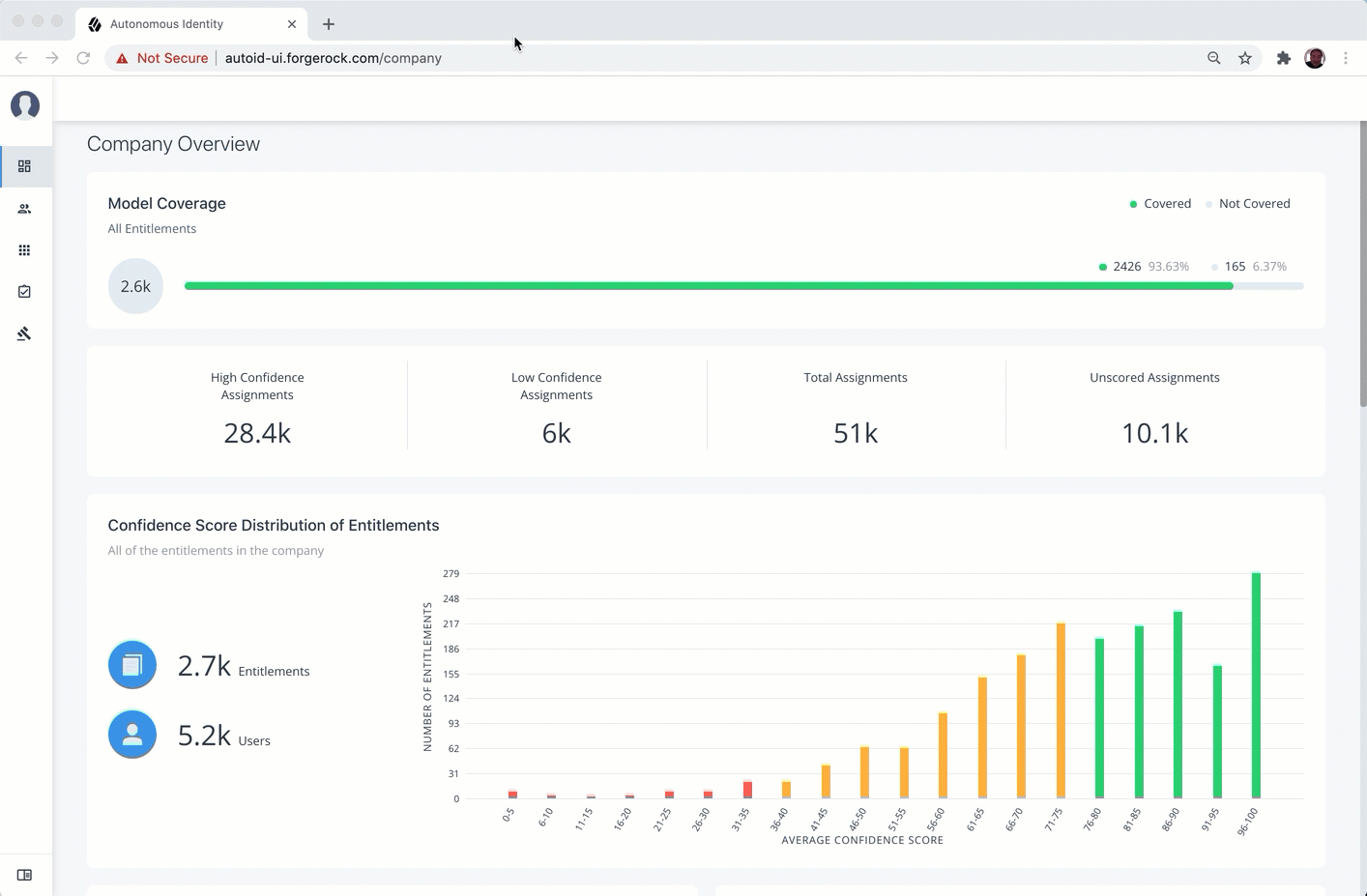
-
On the Swagger page, scroll down to the Login API.
-
In the Login API section, click POST, and then click Try it out.
-
In the request body, enter the username and password of a user. Click Execute.
-
Scroll down to Response Body, and highlight the returned Bearer Token value.
-
Scroll back to the top of the page, and click Authorize. Enter
Bearer <Token Value>
by pasting in the value of the Bearer Token. Click Authorize. You can close the panel.You can now access the Autonomous Identity API endpoints in Swagger.
See it in action
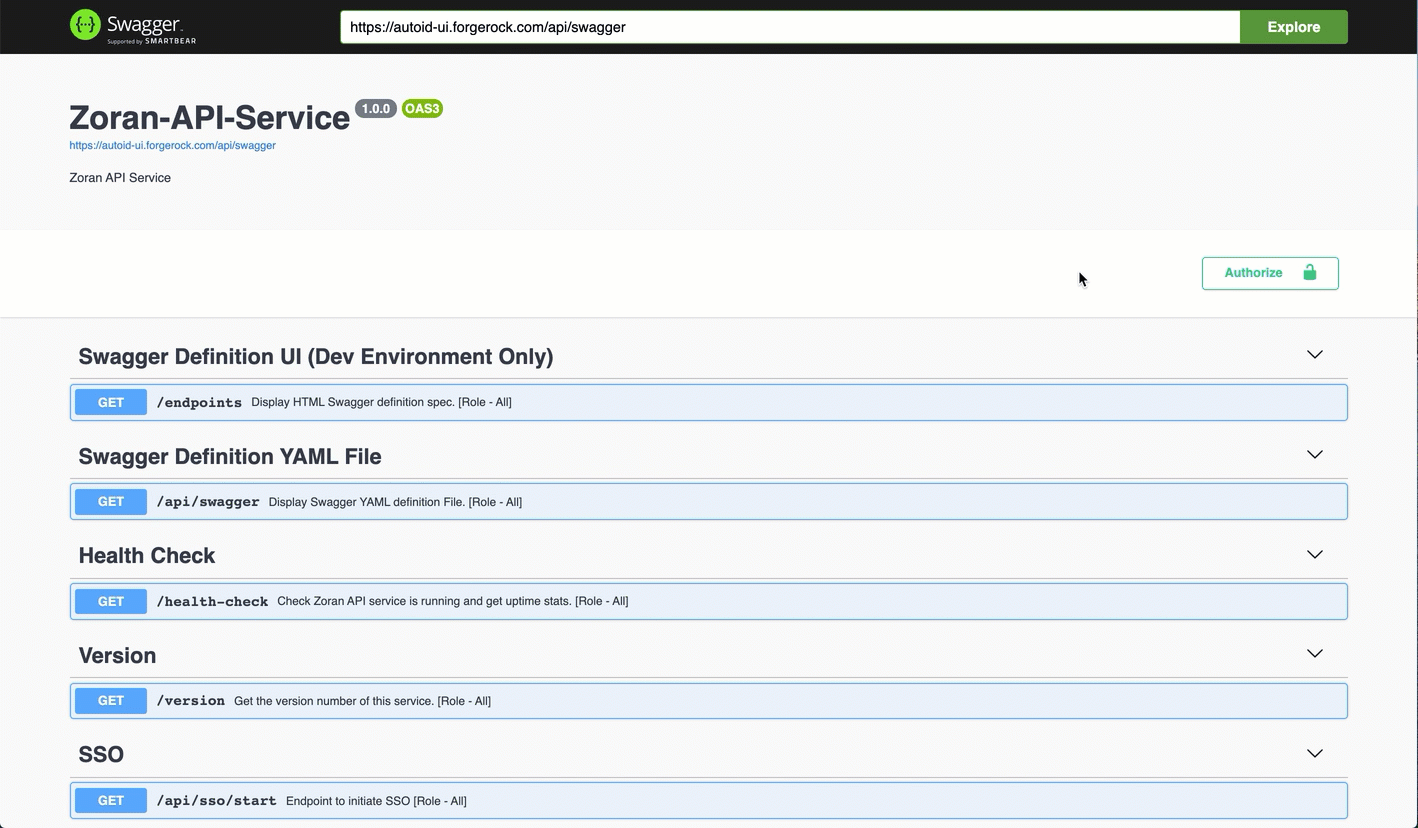
-
Access the Swagger page as presented in Access the Autonomous Identity API on Swagger.
-
Open another browser tab, and point to
https://autoid-ui.forgerock.com/conf/swagger
. You should see a raw test version of the API. -
Go back to the Swagger page in step 1, and enter
https://autoid-ui.forgerock.com/conf/swagger
in the field, and click Explore. You will see the Configuration Service API. -
At the top of the page, click Authorize. Enter
configadmin
and password. The password was set in the~/autoid-config/vault.yml
during install. Click Authorize, and then close the dialog.You can now access the Configuration Service API endpoints in Swagger.
See it in action
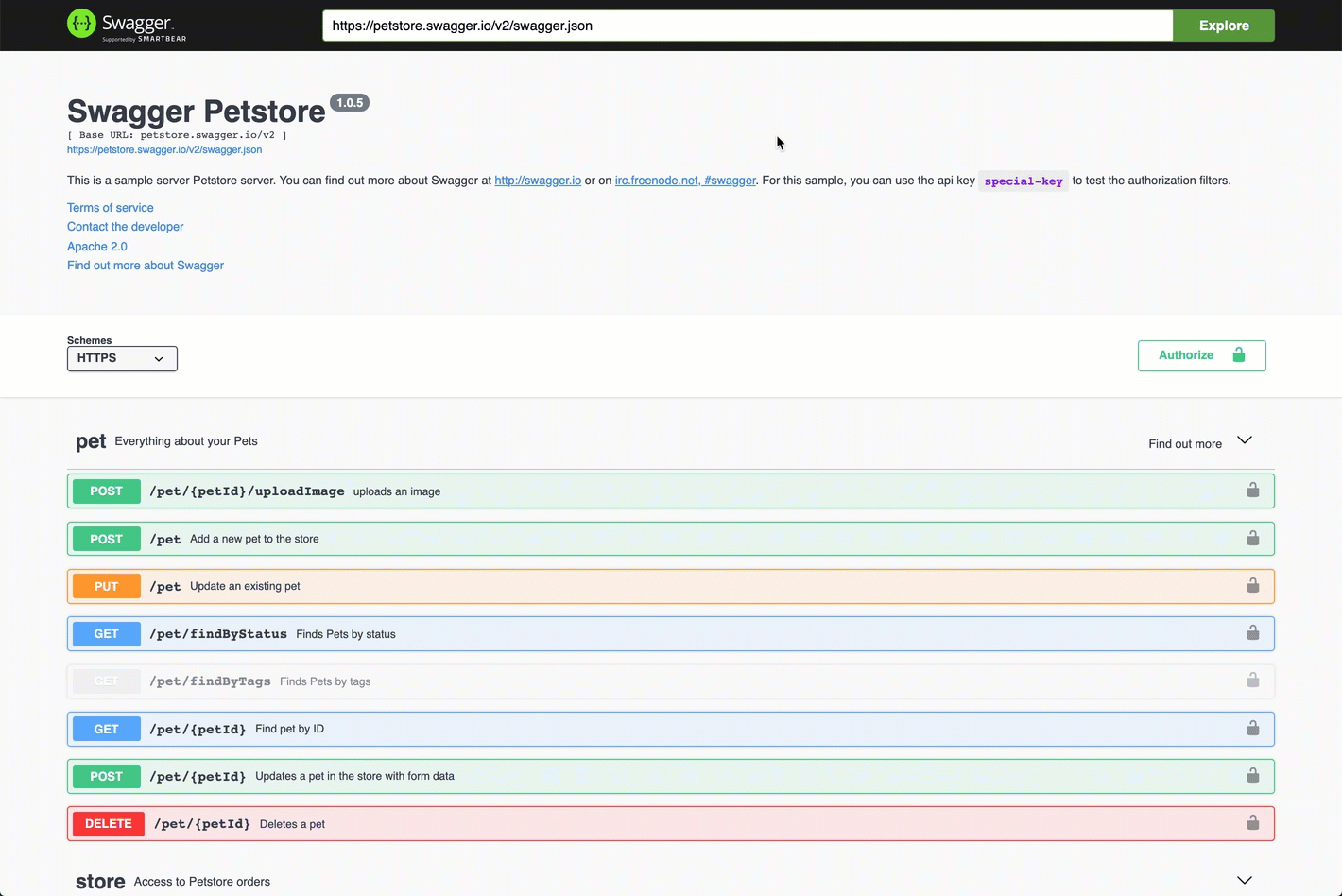
API Service
The following are Autonomous Identity API Service endpoints:
- GET /health-check
-
Check that the Autonomous Identity API service is running. Get uptime statistics. [All]
Endpoint
/health-check
Headers
Content-Type application/json
Body
Example Request
curl -X GET "https://autoid-api.forgerock.com/health-check" \ -H "accept: application/json"
Example Response
{ "status": "zoran-api: OK", "uptime": 5412.465875997, "uptimeFormatted": "1:30:12" }
- GET /version
-
Get the version number of this service. [All]
Endpoint
/version
Headers
Content-Type application/json
Body
Example Request
curl -X GET "https://autoid-api.forgerock.com/version" \ -H "accept: application/json"
Example Response
{ "version": "1.0", }
Authentication
The following are Autonomous Identity authentication endpoints:
- POST /api/authentication/login
-
Log in to the system. The endpoint accepts the
username
andpassword
in the body of the request. The token provided has an expiry date that can be obtained by decoding the returned JWT and using theexp
data inside the token. [All]Endpoint
/api/authentication/login
Headers
Content-Type application/json
Body
{ "username": "admin@test.com", "password": "test" }
Example Request
curl --location --request POST '/api/authentication/login' \ --header 'Content-Type: application/json' \ --data-raw '{ "username": "admin@test.com", "password": "test" }'
Example Response
{ "user": { "dn": "cn=test.user@test.com,dc=example,dc=org", "controls": [], "gidNumber": "7777", "uid": "test.user", "displayName": "Test User", "_groups": [ "Admin" ] }, "token": "123456" }
- POST /api/authentication/renewToken
-
Renew a token for the system. The endpoint accepts the JWT in the header
Authorization: Bearer JWT
. The expiry time of the token is reset and return in the new token. [All]Endpoint
/api/authentication/renewToken
Authorization
Token <token>
Headers
Content-Type application/json
Body
''
Example Request
curl --location --request POST '/api/authentication/renewToken' \ --header 'Content-Type: application/json' \ --data-raw ''
Example Response
{ "user": { "dn": "cn=test.user@test.com,dc=example,dc=org", "controls": [], "gidNumber": "7777", "uid": "test.user", "displayName": "Test User", "_groups": [ "Admin" ] }, "token": "123456" }
- GET /api/authentication/actions
-
Retrieve the permitted actions of the currently authenticated user. [All]
Endpoint
/api/authentication/action
Authorization
Token <token>
Headers
Content-Type application/json
Example Request
curl --location --request GET '/api/authentication/actions' \ --header 'Content-Type: application/json'
Example Response
{ "userActions": [ "*" ], "roleTitle": "Unknown", "homepage": "company" }
SSO
- GET /api/sso/start
-
Endpoint to initiate SSO. [All]
Endpoint
/api/sso/start
Authorization
Token <token>
Headers
Content-Type /
Example Request
curl -X GET "https://autoid-api.forgerock.com/api/sso/start" -H "accept: /"
- GET /api/sso/finish
-
Endpoint to finish SSO. [All]
Endpoint
/api/sso/finish
Authorization
Token <token>
Headers
Content-Type /
Example Request
curl -X GET "https://autoid-api.forgerock.com/api/sso/finish" -H "accept: /"
- GET /api/sso/finish
-
Endpoint to finish SSO. [All]
Endpoint
/api/sso/finish
Authorization
Token <token>
Headers
Content-Type /
Example Request
curl -X GET "https://autoid-api.forgerock.com/api/sso/finish" -H "accept: /"
- GET /api/slo/logout
-
Endpoint to log out of SSO.
Endpoint
/api/slo/logout
Authorization
Token <token>
Headers
Content-Type application/json
Example Request
curl -X GET "https://autoid-api.forgerock.com/api/slo/logout" \ -H "accept: application/json"
Config
The following are Autonomous Identity config endpoint:
- GET /api/config
-
Get the configuration. This endpoint is mainly used by the Autonomous Identity UI microservice to get values stored in Consul. [All]
Endpoint
/api/config
Headers
Content-Type application/json
Example Request
curl -X GET "https://autoid-api.forgerock.com/api/config" \ -H "accept: application/json"
Example Response
{ "thresholds": { "top": 1.01, "high": 0.75, "medium": 0.35, "low": 0, "autoAccess": 0.5 }, "volumeThresholds": { "high": 90, "low": 20 }, "mostAssignedStats": { "count": 100 }, "highVolumeStats": { "high": { "minScore": 0.9, "minUsersCount": 100 }, "low": { "maxScore": 0.2, "minUsersCount": 100 } }, "authorizers": { "ldap": true, "oidc": false } }
- GET /api/admin/reloadUIConfig
-
Reload justification and filterable attributes configuration from JAS. [User, Supervisor, Ent Owner, App Owner, Admin]
Endpoint
/api/admin/reloadUIConfig
Headers
Content-Type /
Example Request
curl -X GET "https://autoid-api.forgerock.com/api/admin/reloadUIConfig" \ -H "accept: /"
Report
Autonomous Identity captures information in its log files that are useful when troubleshooting problems. You can access the reports using REST calls to the Report API endpoint.
- POST /api/report
-
Get reporting data. [All]
Endpoint
/api/report
Authorization
<Bearer Token JWT-value>
Headers
Content-Type application/json
Params
fields
Body
{ "fields": [ "id", "type", "batch_id", "original", "update" ], "reportType": "EventBasedCertification" }
Example Request
curl -X POST "https://autoid-api.forgerock.com/api/report" \ -H "accept: application/json" -H "Content-Type: application/json" \ --data-raw '{ "fields": [ "id", "type", "batch_id", "original", "update" ], "reportType": "EventBasedCertification" }'
Company View
The following are Autonomous Identity company view endpoints:
- GET /api/companyview
-
Get the data for company overview dashboard data. [Executive, Admin]
Endpoint
/api/companyview
Authorization
<Bearer Token JWT-value>
Example Request
curl -X GET "https://autoid-api.forgerock.com/api/companyview" \ -H "accept: application/json"
Example Response
{ "companyView": { "employeeTypes": [ { "type": "Employee", "high": 723, "low": 27, "medium": 1796, "null_conf": 0, "total": 2546 }, { "type": "Non-Employee", "high": 867, "low": 14, "medium": 1768, "null_conf": 0, "total": 2649 } ], "employees_wo_manager": 0, "employees_w_manager": 5200, "entitlements_without_roleowners": 0, "entitlements_with_roleowners": 2456, "total_employees": 5200, "coverage": { "total": 2456, "covered": 2456, "not_covered": 0 }, "entitlementsDistribution": { "no_users": 0, "one_user": 0, "zero_to_five_users": 1, "five_to_ten_users": 1064, "ten_to_hundred_users": 1549, "hundred_to_onek_user": 35, "onek_to_tenk_users": 0, "tenk_users": 0, "hundredk_users": 0 } } }
- GET /api/companyview/allEntitlementsAvgGroups
-
Get the average confidence score list for the company view chart. [Executive, Admin]
Endpoint
/api/companyview/allEntitlementAvgGroups
Authorization
<Bearer Token JWT-value>
Example Request
curl -X GET "https://autoid-api.forgerock.com/api/companyview/allEntitlementAvgGroups" \ -H "accept: application/json"
Example Response
{ "entitlementList": [ { "start": 0, "end": 0.05, "entitlementCount": 2 }, { "start": 0.06, "end": 0.1, "entitlementCount": 14 } ] }
- GET /api/companyview/mostCriticalEntitlements
-
Get the most critical entitlements list. [Executive, Admin]
Endpoint
/api/companyview/mostCriticalEntitlements
Authorization
<Bearer Token JWT-value>
Example Request
curl -X GET "https://autoid-api.forgerock.com/api/companyview/mostCriticalEntitlements" \ -H "accept: application/json"
Example Response
[ { "org": "organization", "entt_id": "ent1", "avg_conf_score": 0.04, "entt_name": "Ent 1", "high": 0, "low": 1, "medium": 0, "seq": 0, "total_employees": 6 }, { "org": "organization", "entt_id": "ent2", "avg_conf_score": 0.04571, "entt_name": "Ent 2", "high": 0, "low": 1, "medium": 0, "seq": 1, "total_employees": 7 } ]
- GET /api/companyview/assignmentStats
-
Get the total assignments, low/high confidence, high volume and low/high confidence, most assigned [Executive, Admin]
Endpoint
/api/companyview/assignmentsStats
Authorization
<Bearer Token JWT-value>
Params
assignmentLimit 1 highVolumeHighMinScore 0.9 highVolumentHighMinUsersCount 100 highVolumenLowMaxScore 0.2 highVolumeLowMinUsersCount 100
Example Request
curl -X GET "https://autoid-api.forgerock.com/api/companyview/assignmentsStats?assignmentsLimit=5" \ -H "accept: application/json"
Example Response
{ "total": 47670, "high": 13145, "low": 4992, "unscored": 4986, "mostAssigned": [ { "count": 344, "entitlement": "ent1" } ], "mostAssignedCount": 35, "highVolume": { "high": 23, "low": 17 } }
- GET /api/companyview/assignmentHistConfSummary/{year}/{month}
-
Get the number of high, medium, and low confidence assignments for the past 12-month period ending in a given year and month. [Executive, admin]
Endpoint
/api/companyview/assignmentsHistConfSummary/2020/01
Authorization
<Bearer Token JWT-value>
Example Request
curl -X GET "https://autoid-api.forgerock.com/api/companyview/assignmentsHistConfSummary/2020/1" \ -H "accept: application/json"
Example Response
[ { "year": 0, "month": 0, "highConf": 0, "medConf": 0, "lowConf": 0, "total": 0 } ]
Single View with Application
This endpoint has been deprecated in this release and will be removed in a future release. |
The following is an Autonomous Identity single view with applications endpoint:
- POST employees
-
Endpoint
/api/singleViewWithApp/employees
Authorization
<Bearer Token JWT-value>
Body
{ "employeeId": "elizabeth.saiz", "includeLastAccessed": true, "pageSize": 5 }
Example Request
curl --location --request POST '/api/singleViewWithApp/employees' \ --header 'Content-Type: application/json' \ --data-raw '{ "employeeId": "elizabeth.saiz", "pageSize": 2, "lastEntitlementId": "0ff681de-ee83-4ab1-82b5-d1cd754a7e28" }'
Example Response
{ "high": 0, "medium": 1, "low": 1, "avg_score": 0.25, "app_name": "", "app_id": "", "entitlement_name": "", "high_risk": null, "userEntt": [ { "user": "elizabeth.saiz", "entitlement": "192aed21-a7d1-40c3-87a3-9dfa4a3d21f5", "app_id": "null", "app_name": "test3", "entitlement_name": "null", "freq": null, "frequnion": null, "high_risk": "null", "justification": [], "score": 0.1, "user_name": "alpha" }, { "user": "elizabeth.saiz", "entitlement": "36bad416-d42c-47c2-991e-623aa3833028", "app_id": "null", "app_name": "test6", "entitlement_name": "null", "freq": null, "frequnion": null, "high_risk": "null", "justification": [], "score": 0.4, "user_name": "vce" } ], "user": "elizabeth.saiz", "entitlementsCount": 14, "entitlementsRemainingCount": 10, "lastEntitlementId": "36bad416-d42c-47c2-991e-623aa3833028" }
User Details
The following are Autonomous Identity user details endpoints:
- POST /api/userDetails
-
Get employee details for Identities views. [User, Supervisor, Ent Owner, App Owner, Admin]
Endpoint
/api/userDetails
Authorization
<Bearer Token JWT-value>
Headers
Content-Type application/json
Body
{ "employeeId": "john.doe", "sortDir": "asc, desc", "lastEntitlementId": "Web_NAS_Share_Case Management_7HQ", "lastRecommendedEnttId": "string" }
Example Request
curl -X POST "https://autoid-api.forgerock.com/api/userDetails" \ -H "accept: application/json" -H "Content-Type: application/json" \ --data-raw '{ "employeeId": "john.doe", "sortDir": "asc, desc", "lastEntitlementId": "Web_NAS_Share_Case Management_7HQ", "lastRecommendedEnttId": "string" }'
Example Response
{ "recommendedEntt": { "predictions": [ { "usr_key": "john.doe", "ent": "ent1", "conf": "0.88", "freq": "10.0", "frequnion": "9", "rule": [ { "title": "Chief", "value": "Yes" }, { "title": "Employee Type", "value": "Employee" } ], "entt": { "entitlement": "Ent 1", "app_id": "app1", "role": "role.owner", "app_name": "App 1", "entitlement_name": "Ent 1", "high_risk": "High", "roleOwnerDisplayName": "Role Owner", "requestorCanAccess": false } }, { "usr_key": "john.doe", "ent": "ent2", "conf": "1.00", "freq": "4.0", "frequnion": "4", "rule": [ { "title": "Job Code Name", "value": "Business Representitive" }, { "title": "Line of Business", "value": "Portfolio Management" }, { "title": "Department", "value": " South" }, { "title": "Employee Type", "value": "Employee" } ], "entt": { "entitlement": "ent2", "app_id": "app1", "role": "role.owner", "app_name": "App 1", "entitlement_name": "Ent 2", "high_risk": "High", "roleOwnerDisplayName": "Role Owner", "requestorCanAccess": true } } ], "entitlementsCount": 14, "entitlementsRemainingCount": 9, "lastEntitlementId": "ent2" }, "userEntt": [ { "user": "john.doe", "entitlement": "ent3", "app_id": "app1", "app_name": "App 1", "entitlement_name": "Ent 3", "freq": "10.0", "frequnion": "9", "high_risk": "High", "justification": [ { "title": "Chief", "value": "Yes" }, { "title": "Employee Type", "value": "Employee" } ], "score": 0.88, "user_name": "John Doe", "lastAccessed": "2020-01-01 00:00:00", "requestorCanAccess": false, "rawJustification": [ "CHIEF_YES_NO_Yes", "USR_EMP_TYPE_Employee" ] }, { "user": "john.doe", "entitlement": "ent4", "app_id": "app1", "app_name": "App 1", "entitlement_name": "Ent 4", "freq": "4.0", "frequnion": "4", "high_risk": "High", "justification": [ { "title": "Job Code Name", "value": "Business Representitive" }, { "title": "Line of Business", "value": "Portfolio Management" }, { "title": "Department", "value": " South" }, { "title": "Employee Type", "value": "Employee" } ], "score": 1, "user_name": "John Doe", "lastAccessed": "2020-01-01 00:00:00", "requestorCanAccess": false, "rawJustification": [ "JOBCODE_NAME_Business Representitive", "LINE_OF_BUSINESS_Portfolio Management", "USR_DEPARTMENT_NAME_Customer Operations_ South", "USR_EMP_TYPE_Employee" ] } ], "user": { "displayName": "John Doe", "hrData": [ { "title": "Job Code Name", "id": "JOBCODENAME", "value": "Business Representitive" }, { "title": "Line of Business", "id": "LINEOFBUSINESS", "value": "Portfolio Management" }, { "title": "Department", "id": "DEPARTMENT", "value": " South" }, { "title": "Employee Type", "id": "EMPTYPE", "value": "Employee" } ] }, "entitlementsCount": 2, "entitlementsRemainingCount": 9, "lastEntitlementId": "ent4" }
- POST /api/userDetails/hrData
-
Get a user’s HR data. [User, Supervisor, Ent Owner, App Owner, Admin]
Endpoint
/api/userDetails/hrData
Authorization
<Bearer Token JWT-value>
Headers
Content-Type application/json
Body
{ "employeeId": "john.doe" }
Example Request
curl -X POST "https://autoid-api.forgerock.com/api/userDetails/hrData" \ -H "accept: application/json" -H "Content-Type: application/json" \ --data-raw '{ "employeeId": "john.doe" }'
Example Response
{ "user": [ { "id": "USER_NAME", "title": "User Name", "value": "john.doe" }, { "id": "CHIEF", "title": "Chief", "value": "Yes" }, { "id": "CITY", "title": "City", "value": "Toledo" }, { "id": "USER_DISPLAY_NAME", "title": "User Display Name", "value": "John Doe" }, { "id": "EMPLOYEE_TYPE", "title": "Employee Type", "value": "Employee" }, { "id": "MANAGER", "title": "Manager", "value": "the.manager" } ], "displayName": "John Doe" }
- POST /api/userDetails/search
-
Search for users by name and with applied filters. [Executive, Supervisor, App Owner, Admin]
Endpoint
/api/userDetails/search
Authorization
<Bearer Token JWT-value>
Headers
Content-Type application/json
Body
{ "username": "john.doe" }
Example Request
curl -X POST "https://autoid-api.forgerock.com/api/userDetails/search" \ -H "accept: application/json" -H "Content-Type: application/json" \ --data-raw '{ "username": "john.doe" }'
Example Response
{ "values": [ { "user": "john.doe", "isapplicationowner": "false", "isentitlementowner": "false", "issupervisor": "false", "userdisplayname": "John Doe" } ] }
- POST /api/userDetails/ent/autoprovision
-
Get user’s entitlements for autoprovisioning. [Admin]
Endpoint
/api/userDetails/ent/autoprovision
Authorization
<Bearer Token JWT-value>
Headers
Content-Type application/json
Body
{ "user": "john.doe" }
Example Request
curl -X POST "https://autoid-api.forgerock.com/api/userDetails/ent/autoprovision" \ -H "accept: application/json" -H "Content-Type: application/json" \ --data-raw '{ "user": "john.doe" }'
Example Response
{ "usr_id": "string", "usr_name": "string", "ents": [ { "ent_id": "string", "ent_name": "string", "ent_attribute": "string", "ent_risk_level": "string", "score": 0, "freq": 0, "frequnion": 0, "justification": [ { "title": "string", "value": "string" } ], "app_id": "string", "app_name": "string" } ], "cursor": "string" } No links
- POST /api/userDetails/autoAction
-
Get the list of entitlements for a user or list of users for an entitlement to provision, revoke, or certify. [Admin]
Endpoint
/api/userDetails/autoAction
Authorization
<Bearer Token JWT-value>
Headers
Content-Type application/json
Body
{ "action": "addAccess", "usrId": "john.doe", "entId": "entitlement_1", "thresholds": { "gte": 0, "gt": 0, "lte": 0, "lt": 0 }, "cursor": "string" }
Example Request
curl -X POST "https://autoid-api.forgerock.com/api/userDetails/autoAction" \ -H "accept: application/json" -H "Content-Type: application/json" \ --data-raw '{ "action": "addAccess", "usrId": "john.doe", "entId": "entitlement_1", "thresholds": { "gte": 0, "gt": 0, "lte": 0, "lt": 0 }, "cursor": "string" }'
- GET /api/userDetails/drivingFactor
-
Get the driving factor data. [User, Supervisor, Ent Owner, App Owner, Admin]
Endpoint
/api/userDetails/drivingFactor
Authorization
<Bearer Token JWT-value>
Headers
Content-Type application/json
Params
{ "entitlement": "entitlement1" }
Example Request
curl -X POST "https://autoid-api.forgerock.com/api/userDetails/drivingFactor" \ -H "accept: application/json" -H "Content-Type: application/json" \ --data-raw '{ "entitlement": "entitlement1" }'
Example Request
[ { "ent": "ent1", "attribute": { "title": "Chief", "value": "No" }, "count": 3, "rawAttribute": "CHIEF_YES_NO_No" }, { "ent": "ent1", "attribute": { "title": "City", "value": "Tacoma" }, "count": 5, "rawAttribute": "CITY_Tacoma" } ]
Access Control
The following are Autonomous Identity access control endpoints:
- POST /api/userDetails/decisions
-
Get the current entitlement decisions for the user. [Supervisor, Ent Owner, App Owner, Admin]
Endpoint
/api/userDetails/decisionsl
Authorization
<Bearer Token JWT-value>
Param
user=john.doe
Example Request
curl -X GET "https://autoid-api.forgerock.com/api/userDetails/decisions?user=john.doe" \ -H "accept: application/json"
Example Response
{ "decisions": [ { "entitlement": "string", "is_certified": true, "is_revoked": true, "is_processed": true, "is_archived": true, "author": "string", "author_name": "string", "author_type": "string", "reason": "string", "last_updated": "2021-04-14T18:45:46.916Z" } ] }
- POST /api/userDetails/decisions
-
Update entitlement decisions for users. [Supervisor, Ent Owner, App Owner, Admin]
Endpoint
/api/userDetails/decisions
Authorization
<Bearer Token JWT-value>
Body
{ "assignments": [ { "user": "string", "entitlements": [ "string" ] } ], "is_certified": true, "is_revoked": true, "is_requested": true, "is_processed": true, "reason": "string" }
Example Request
curl -X POST "https://autoid-api.forgerock.com/api/userDetails/decisions" \ -H "accept: /" -H "Content-Type: application/json" \ --data-raw '{ "assignments": [ { "user": "string", "entitlements": [ "string" ] } ], "is_certified": true, "is_revoked": true, "is_requested": true, "is_processed": true, "reason": "string" }'
- POST /api/rules/decision
-
Update rule decisions. [Supervisor, Ent Owner, App Owner, Admin]
Endpoint
/api/rules/decision
Authorization
<Bearer Token JWT-value>
Body
{ "rules": [ { "entitlement": "string", "justification": [ "string" ] } ], "is_autocertify": true, "is_autorequest": true, "autocertify_reason": "string", "autorequest_reason": "string" }
Example Request
curl -X POST "https://autoid-api.forgerock.com/api/rules/decision" \ -H "accept: /" -H "Content-Type: application/json" \ --data-raw '{ "rules": [ { "entitlement": "string", "justification": [ "string" ] } ], "is_autocertify": true, "is_autorequest": true, "autocertify_reason": "string", "autorequest_reason": "string" }'
Applications
The following are Autonomous Identity applications view endpoints:
- GET /api/applications
-
Get a list of applications and stats for an Application Owner. [App Owner, Admin]
Endpoint
/api/applications
Authorization
<Bearer Token JWT-value>
Params
ownerId (optional) derick.hui cursor (optional) string (Indicator on where to start a 2+ page list)
Example Request
curl -X GET "https://autoid-api.forgerock.com/api/applications?ownerId=derick.hui" \ -H "accept: application/json"
Example Response
{ "cursor": "string", "total_applications": 0, "total_entitlements": 0, "total_assignments": 0, "applications": [ { "app_id": "string", "app_name": "string", "high": 0, "medium": 0, "low": 0, "avg": 0 } ] }
- POST /api/applications/{appId}
-
Get a list of entitlements and stats for a selected application. [App Owner, Admin]
Endpoint
/api/applications/{appId}
Authorization
<Bearer Token JWT-value>
Params
appId (required) app_1 cursor (optional) string (Indicator on where to start a 2+ page list)
Body
{ "filters": [ { "type": "user", "attribute": "city", "value": ["Seattle", "Denver"] }, { "type": "user", "attribute": "line_of_business", "value": ["Distribution Operations"] } ] }
Example Request
curl -X POST "https://autoid-api.forgerock.com/api/applications/app_1" \ -H "accept: application/json" -H "Content-Type: application/json" \ --data-raw '{ "filters": [ { "type": "user", "attribute": "city", "value": ["Seattle", "Denver"] }, { "type": "user", "attribute": "line_of_business", "value": ["Distribution Operations"] } ] }'
Example Response
{ "cursor": "string", "total_entitlements": 0, "total_users": 0, "total_rules": 0, "entitlements": [ { "ent": "string", "ent_name": "string", "high": 0, "medium": 0, "low": 0, "avg": 0 } ] }
- POST /api/applications/{appId}/assignments
-
Get filterable user-entitlement assignment and decision data for a specific application. [App Owner, Admin]
Endpoint
api/applications/{appId}/assignments
Authorization
<Bearer Token JWT-value>
Params
appId (required) app_1 user string cursor (optional) string (Indicator on where to start a 2+ page list) sortBy string sortDir string
Body
{ "filters": [ { "type": "user", "attribute": "city", "value": [ "Seattle", "Denver" ] }, { "type": "user", "attribute": "line_of_business", "value": [ "Distribution Operations" ] } ] }
Example Request
curl -X POST "https://autoid-api.forgerock.com/api/applications/app_1/assignments" \ -H "accept: application/json" -H "Content-Type: application/json" \ --data-raw '{ "filters": [ { "type": "user", "attribute": "city", "value": [ "Seattle", "Denver" ] }, { "type": "user", "attribute": "line_of_business", "value": [ "Distribution Operations" ] } ] }'
Example Response
{ "cursor": "string", "total_users": 0, "total_entitlements": 0, "total_assignments": 0, "assignments": [ { "ent": "string", "ent_name": "string", "confidence": 0, "user_id": "string", "user_name": "string", "isCertified": true, "dateCertified": "2021-04-14T19:10:39.178Z", "isRevoked": true, "dateRevoked": "2021-04-14T19:10:39.178Z", "isRequested": true, "dateRequested": "2021-04-14T19:10:39.178Z", "isProcessed": true, "approvalAuthor": { "id": "string", "name": "string" } } ] }
- GET /api/applications/search
-
Search all applications. [App Owner, Admin]
Endpoint
/api/applications/search
Authorization
<Bearer Token JWT-value>
Params
by appOwner or enttOwner user user ID q Search query string
Example Request
curl -X GET "https://autoid-api.forgerock.com/api/applications/search" \ -H "accept: application/json"
Example Response
{ "values": [ { "app_id": "string", "app_name": "string" } ] }
Entitlements
The following are Autonomous Identity filtering by entitlements endpoints:
- GET /api/entitlements/search
-
Search for entitlements by name and with applied filters. [Ent Owner, App Owner, Admin]
Endpoint
/api/entitlements/search?q=QueryString
Authorization
<Bearer Token JWT-value>
Params
by appOwner or enttOwner user user ID q Search query string (required) appId Application ID to use as a filter
Example Request
curl --location --request GET 'https://autoid-api.forgerock.com/api/entitlements/search?by=enttOwner&user=john.doe&q=WEB&appId=Salesforce' \ --header 'Content-Type: application/json'
Example Response
{ "values": [ { "id": "string", "app_id": "string", "app_name": "string", "entt_name": "string" } ] }
- POST /api/entitlements/stats
-
Get data for entitlements view. [Supervisor, Ent Owner, Admin]
Endpoint
/api/entitlements/stats?by=supervisor/entitlementOwner/admin
Authorization
<Bearer Token JWT-value>
Params
by supervisor, roleOwner
Body
{ "ownerId": "timothy.slack", "isHighRiskOnly": true, "isMediumLowRiskOnly": false, "isUserEntitlementsIncluded": true, "filters": [{ "type": "app_id", "group": "criticality", "value": "Essential" }] }
Example Request
curl --location --request POST 'https://autoid-api.forgerock.com/api/entitlements/stats?by=supervisor' \ --header 'content-type: application/json' \ --data-raw '{ "ownerId": "timothy.slack", "isHighRiskOnly": true, "isMediumLowRiskOnly": false, "isUserEntitlementsIncluded": true, "filters": [{ "type": "app_id", "group": "criticality", "value": "Essential" }] }'
Example Response
{ "total_entitlements": 0, "total_subordinates": 0, "unscoredEntitlements": 0, "scoredEntitlements": 0, "usersWithNoEntitlement": 0, "usersWithNoScoredEntitlement": 0, "distinct_apps": [ { "app_id": "string", "app_name": "string", "low": 0, "medium": 0, "high": 0 } ], "users": [ { "user": "string", "user_name": "string", "high": 0, "medium": 0, "low": 0, "avg": "string" } ], "entitlements": [ { "entitlement": "string", "entitlement_name": "string", "app_id": "string", "high_risk": "string", "high": 0, "medium": 0, "low": 0, "avg": "string" } ] }
- GET /api/entitlements/id/{id}
-
Get entitlement details. [User, Supervisor, Ent Owner, App Owner, Admin]
Endpoint
/api/entitlements/id/{id+}
Authorization
<Bearer Token JWT-value>
Params
by entitlement ID
Example Request
curl -X GET "https://autoid-api.forgerock.com/api/entitlements/id/1234" \ -H "accept: application/json"
Example Response
{ "entitlement_name": "string", "scores": { "avg": 0, "high": 0, "medium": 0, "low": 0 }, "drivingFactors": [ { "attribute": { "id": "string", "title": "string", "value": "string" }, "count": 0 } ], "userScores": [ { "score": 0, "count": 0 } ], "users": [ { "user": "string", "user_name": "string", "app_id": "string", "freq": 0, "frequnion": 0, "justification": [ { "title": "string", "value": "string" } ], "rawJustification": [ "string" ], "score": 0 } ] }
- GET /api/entitlements/unscored
-
Get unscored entitlements and users for a given Supervisor or Entitlement Owner ID. [Supervisor, Ent Owner, Admin]
Endpoint
/api/entitlements/unscored
Authorization
<Bearer Token JWT-value>
Params
by supervisor, entitlement owner user supervisor or entitlement owner user ID
Example Request
curl -X GET "https://autoid-api.forgerock.com/api/entitlements/unscored?by=supervisor&user=1234" \ -H "accept: application/json"
Rules
The following are Autonomous Identity rules endpoints:
- GET Rule Stats
-
List information and statistics regarding available rules. [Ent Owner, App Owner, Admin]
Endpoint
/api/rules/info
Authorization
<Bearer Token JWT-value>
Params
by enttowner, appOwner user patrick.murphy
Example Request
curl -X GET "https://autoid-api.forgerock.com/api/rules/info?by=appOwner&user=patrick.murphy" \ -H "accept: application/json"
Example Response
{ "countRules": 0, "countAssignments": 0, "countApplications": 0, "applications": [ { "app_id": "string", "app_name": "string", "countAssignments": 0, "low": 0, "medium": 0, "high": 0 } ] }
- GET /api/rules
-
List the available rules for a user. [Ento Owner, App Owner, Admin]
Endpoint
/api/rules/
Authorization
<Bearer Token JWT-value>
Params
by enttOwner, appOwner user david.elliott cursor (indicator on where to start a 2+ page list) filter (filters to apply when searching) pageSize (number of records per page to retrieve)
Example Request
curl -X GET "lowConfidence=true&filter[medConfidence]=true&filter[highConfidence]=true&filter[autoCertify]=true&filter[autoRequest]=true&filter[entitlement]=string" \ -H "accept: application/json"
Example Response
{ "cursor": "string", "rules": [ { "entitlement": { "owner": "string", "ent": "string", "ent_name": "string" }, "app": { "app_id": "string", "app_name": "string", "ent": "string" }, "justification": [ { "id": "string", "title": "string", "value": "string" } ], "assignees": [ { "id": "string", "name": "string", "properties": [ { "id": "string", "title": "string", "value": "string" } ], "last_usage": "2021-04-14T19:40:27.740Z" } ], "confidence": 0, "countUnassigned": 0, "countAssigned": 0, "isAutoCertify": true, "autoCertifyDate": "2021-04-14T19:40:27.740Z", "isAutoRequest": true, "autoRequestDate": "2021-04-14T19:40:27.740Z", "approvalAuthor": { "id": "string", "name": "string" }, "requestApprovalReason": "string", "certifyApprovalReason": "string" } ] }
Filters
The following are Autonomous Identity Filters endpoints:
- GET /api/filters/owner
-
Get filterable attributes and values. [Supervisor, Ent Owner, Admin]
Endpoint
/api/filters/owner?by=supervisor&user=albert.pardini
Authorization
<Bearer Token JWT-value>
Query Parameters
by supervisor, enttOwner user albert.pardini
Example Request
curl -X GET "https://autoid-api.forgerock.com/api/filters/owner?by=supervisor&user=albert.pardini" \ -H "accept: application/json"
Example Response
{ "items": [ { "title": "string", "field": "string", "filters": { "field": "string", "title": "string", "options": [ { "text": "string", "value": "string", "count": 0 } ] } } ] }
- GET /api/filters/app
-
Get filterable attributes and values. [App Owner, Admin]
Endpoint
/api/filters/app
Authorization
<Bearer Token JWT-value>
Query Parameters
id: application ID
Example Request
curl -X GET "https://autoid-api.forgerock.com/api/filters/app?id=app_1" \ -H "accept: application/json"
Example Response
{ "items": [ { "title": "string", "field": "string", "filters": { "field": "string", "title": "string", "options": [ { "text": "string", "value": "string", "count": 0 } ] } } ] }