Default policy for managed objects
Policies applied to managed objects are configured in two places:
-
A policy script that defines each policy and specifies how policy validation is performed.
For more information, refer to Policy Script.
-
A managed object policy element, defined in your managed object configuration, that specifies which policies are applicable to each managed resource. For more information, refer to Policy Configuration Element.
The policy configuration determines which policies apply to resources other than managed objects. The default policy configuration includes policies that are applied to internal user objects, but you can extend the configuration to apply policies to system objects.
Policy script
The policy script file (openidm/bin/defaults/script/policy.js
) separates policy configuration into two parts:
-
A policy configuration object, which defines each element of the policy. For more information, refer to Policy Configuration Objects.
-
A policy implementation function, which describes the requirements that are enforced by that policy.
Together, the configuration object and the implementation function determine whether an object is valid in terms of the applied policy. The following excerpt of a policy script file configures a policy that specifies that the value of a property must contain a certain number of capital letters:
...
{ "policyId": "at-least-X-capitals",
"policyExec": "atLeastXCapitalLetters",
"clientValidation": true,
"validateOnlyIfPresent": true,
"policyRequirements": ["AT_LEAST_X_CAPITAL_LETTERS"]
},
...
policyFunctions.atLeastXCapitalLetters = function(fullObject, value, params, property) {
var isRequired = _.find(this.failedPolicyRequirements, function (fpr) {
return fpr.policyRequirement === "REQUIRED";
}),
isString = (typeof(value) === "string"),
valuePassesRegexp = (function (v) {
var test = isString ? v.match(/[A-Z]/g) : null;
return test !== null && test.length >= params.numCaps;
}(value));
if ((isRequired || isString) && !valuePassesRegexp) {
return [ { "policyRequirement" : "AT_LEAST_X_CAPITAL_LETTERS", "params" : {"numCaps": params.numCaps} } ];
}
return [];
}
...
To enforce user passwords that contain at least one capital letter, the policyId
from the preceding example is applied to the appropriate resource (managed/user/*
). The required number of capital letters is defined in the policy configuration element of the managed object configuration file (see Policy Configuration Element).
Policy configuration objects
Each element of the policy is defined in a policy configuration object. The structure of a policy configuration object is as follows:
{
"policyId": "minimum-length",
"policyExec": "minLength",
"clientValidation": true,
"validateOnlyIfPresent": true,
"policyRequirements": ["MIN_LENGTH"]
}
|
A unique ID that enables the policy to be referenced by component objects. |
|
The name of the function that contains the policy implementation. For more information, refer to Policy Implementation Functions. |
|
Indicates whether the policy decision can be made on the client. When |
|
Notes that the policy is to be validated only if the field within the object being validated exists. |
|
An array containing the policy requirement ID of each requirement that is associated with the policy. Typically, a policy will validate only one requirement, but it can validate more than one. |
Policy implementation functions
Each policy ID has a corresponding policy implementation function that performs the validation. Implementation functions take the following form:
function <name>(fullObject, value, params, propName) {
<implementation_logic>
}
-
fullObject
is the full resource object that is supplied with the request. -
value
is the value of the property that is being validated. -
params
refers to theparams
array that is specified in the property’s policy configuration. -
propName
is the name of the property that is being validated.
The following example shows the implementation function for the required
policy:
function required(fullObject, value, params, propName) {
if (value === undefined) {
return [ { "policyRequirement" : "REQUIRED" } ];
}
return [];
}
Default policy reference
IDM includes the following default policies and parameters:
Policy Id | Parameters | |
---|---|---|
The property is required; not optional. |
||
The property can’t be empty. |
||
The property can’t be null. |
||
The property must be unique. |
||
Tests for uniqueness and internal user conflicts. |
||
Tests for internal user conflicts. |
||
Matches a regular expression. |
|
The regular expression pattern. |
Tests for the specified types. |
|
|
Tests for a valid query filter. |
||
Tests for valid array items. |
||
Tests for a valid date. |
||
Tests for a valid date format. |
||
Tests for a valid time. |
||
Tests for a valid date and time. |
||
Tests for a valid duration format. |
||
Tests for a valid email address. |
||
Tests for a valid name format. |
||
Tests for a valid phone number format. |
||
The property must contain the minimum specified number of capital letters. |
|
Minimum number of capital letters. |
The property must contain the minimum specified number of numbers. |
|
Minimum number of numbers. |
Tests for a valid number. |
||
The property value must be greater than the |
|
The minimum value. |
The property value must be less than the |
|
The maximum value. |
The property’s minimum string length. |
|
The minimum string length. |
The property’s maximum string length. |
|
The maximum string length. |
The property cannot contain values of the specified fields. |
|
A comma-separated list of the fields to check against. For example, the default managed user password policy specifies |
The property cannot contain the specified characters. |
|
A comma-separated list of disallowed characters. For example, the default managed user |
The property cannot contain duplicate characters. |
||
A sync mapping must exist for the property. |
||
Tests for valid permissions. |
||
Tests for valid access flags. |
||
Tests for a valid privilege path. |
||
Tests for valid temporal constraints. |
Policy configuration element
Properties defined in the managed object configuration can include a policies
element that specifies how policy validation should be applied to that property. The following excerpt of the default managed object configuration shows how policy validation is applied to the password
and _id
properties of a managed/user object.
You can only declare policies on top-level managed object attributes. Nested attributes (those within an array or object) cannot have policy declared on them. |
{
"name" : "user",
"schema" : {
"id" : "http://jsonschema.net",
"properties" : {
"_id" : {
"description" : "User ID",
"type" : "string",
"viewable" : false,
"searchable" : false,
"userEditable" : false,
"usageDescription" : "",
"isPersonal" : false,
"policies" : [
{
"policyId" : "cannot-contain-characters",
"params" : {
"forbiddenChars" : [
"/"
]
}
}
]
},
"password" : {
"title" : "Password",
"description" : "Password",
"type" : "string",
"viewable" : false,
"searchable" : false,
"userEditable" : true,
"encryption" : {
"purpose" : "idm.password.encryption"
},
"scope" : "private",
"isProtected": true,
"usageDescription" : "",
"isPersonal" : false,
"policies" : [
{
"policyId" : "minimum-length",
"params" : {
"minLength" : 8
}
},
{
"policyId" : "at-least-X-capitals",
"params" : {
"numCaps" : 1
}
},
{
"policyId" : "at-least-X-numbers",
"params" : {
"numNums" : 1
}
},
{
"policyId" : "cannot-contain-others",
"params" : {
"disallowedFields" : [
"userName",
"givenName",
"sn"
]
}
}
]
}
}
}
}
Note that the policy for the _id
property references the function cannot-contain-characters
, that is defined in the policy.js
file. The policy for the password
property references the functions minimum-length
, at-least-X-capitals
, at-least-X-numbers
, and cannot-contain-others
, that are defined in the policy.js
file. The parameters that are passed to these functions (number of capitals required, and so forth) are specified in the same element.
Validate managed object data types
The type
property of a managed object specifies the data type of that property, for example, array
, boolean
, number
, null
, object
, or string
. For more information about data types, refer to the JSON Schema Primitive Types section of the JSON Schema standard.
The type
property is subject to policy validation when a managed object is created or updated. Validation fails if data does not match the specified type
, such as when the data is an array
instead of a string
. The default valid-type
policy enforces the match between property values and the type
defined in the managed object configuration.
IDM supports multiple valid property types. For example, you might have a scenario where a managed user can have more than one telephone number, or a null telephone number (when the user entry is first created and the telephone number is not yet known). In such a case, you could specify the accepted property type as follows in your managed object configuration:
"telephoneNumber" : {
"type" : "string",
"title" : "Telephone Number",
"description" : "Telephone Number",
"viewable" : true,
"userEditable" : true,
"pattern" : "^\\+?([0-9\\- \\(\\)])*$",
"usageDescription" : "",
"isPersonal" : true,
"policies" : [
{
"policyId" : "minimum-length",
"params" : {
"minLength" : 1
}
},
{
"policyId": "maximum-length",
"params": {
"maxLength": 255
}
}
]
}
In this case, the valid-type
policy from the policy.js
file checks the telephone number for an accepted type
and pattern
, either for a real telephone number or a null
entry.
Configure policy validation using the admin UI
To configure policy validation for a managed object type using the admin UI, update the configuration of the object type—a high-level overview:
-
Go to the managed object, and edit or create a property.
-
Click the Validation tab, and add the policy.
Show Me
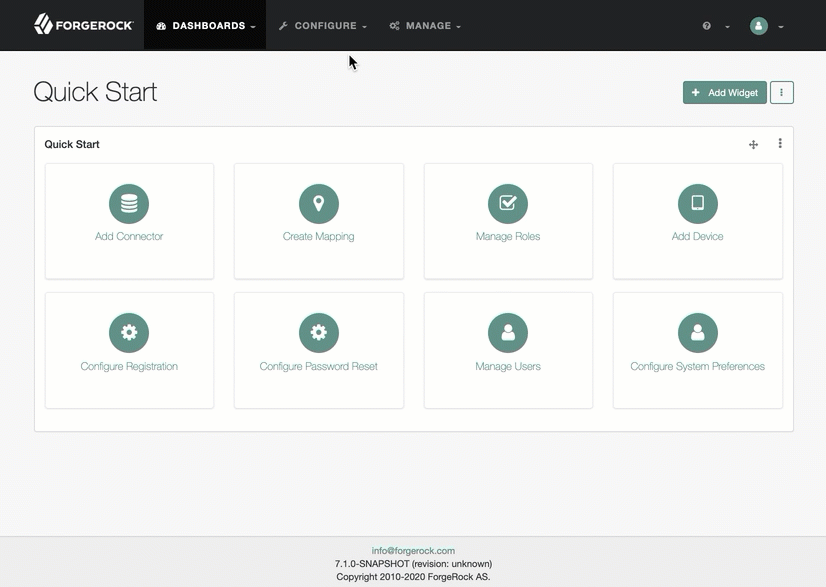
-
From the navigation bar, click Configure > Managed Objects.
-
On the Managed Objects page, edit or create a managed object.
-
On the Managed Object NAME page, do one of the following:
-
To edit an existing property, click the property.
-
To create a property, click Add a Property, enter the required information, and click Save.
-
Now click the property.
-
-
-
From the Validation tab, click Add Policy.
-
In the Add/Edit Policy window, enter information in the following fields, and click Add or Save:
- Policy Id
-
Refers to the unique
PolicyId
in thepolicy.js
file.For a list of the default policies, refer to Policy Reference.
- Parameter Name
-
Refers to the parameters for the
PolicyId
. For a list of the default policy parameters, refer to Policy Reference. - Value
-
The parameter’s value to validate.
Be cautious when using Validation Policies. If a policy relates to an array of relationships, such as between a user and multiple devices, Return by Default should always be set to |