Authenticate by using a WebAuthn device
After the user registers their mobile device they can use it as an authenticator, with its registered key pair, through the WebAuthn Authentication node, which the Android SDK returns as a WebAuthnAuthenticationCallback
.
If the device supports passkeys, the operating system displays a list of available passkeys:
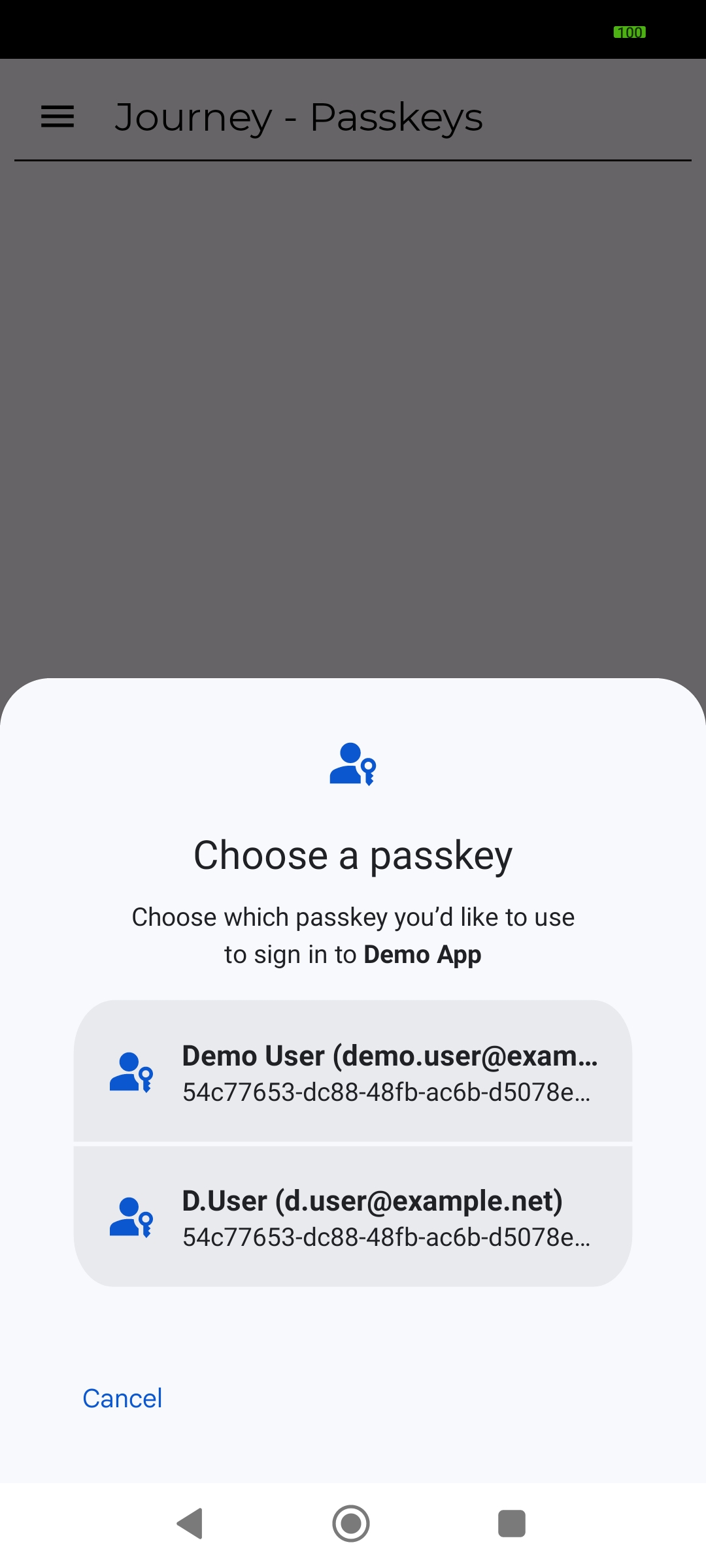
Note that removing credentials stored on the client device does not remove the associated data from the server. You will need to register the device again after removing credentials from the client.
As part of authentication process, the SDK provides the WebAuthnAuthenticationCallback
for authenticating the device as a credential.
WebAuthnAuthenticationCallback callback = node.getCallback(WebAuthnAuthenticationCallback.class);
callback.authenticate(requireContext(), node, webAuthnKeySelector.DEFAULT, new FRListener<Void>() {
@Override
public void onSuccess(Void result) {
// Authentication is successful
// Continue the journey by calling next()
}
@Override
public void onException(Exception e) {
// An error occurred during the authentication process
// Continue the journey by calling next()
}
});
fun WebAuthnAuthenticationCallback(
callback: WebAuthnAuthenticationCallback,
node: Node,
onCompleted: () -> Unit
) {
val context = LocalContext.current
try {
callback.authenticate(context, node)
// Authentication successful
currentOnCompleted()
} catch (e: CancellationException) {
// User cancelled authentication
} catch (e: Exception) {
// An error occurred during the authentication process
currentOnCompleted()
}
}
The If the current node has both |
WebAuthnKeySelector
An optional WebAuthnKeySelector
parameter can be provided for authentication.
The WebAuthnKeySelector.select()
method is invoked
when Username from device
is enabled in the WebAuthn Authentication node.
This feature requires that Username to device
is enabled in the WebAuthn Registration node as well.
With these options enabled, the registered key pair is associated with the username,
and the SDK can present a list of registered keys to the user
to continue the authentication process without collecting a username.
The |
callback.authenticate(this, node, new WebAuthnKeySelector() {
@Override
public void select(@NonNull FragmentManager fragmentManager,
@NonNull List<PublicKeyCredentialSource> sourceList,
@NonNull FRListener<PublicKeyCredentialSource> listener) {
//Always pick the first one.
listener.onSuccess(sourceList.get(0));
}
}, new FRListener<Void>() {
@Override
public void onSuccess(Void result) {
//...
}
@Override
public void onException(Exception e) {
//...
}
});