Build advanced token security in a JavaScript single-page app
This tutorial covers the advanced development required for implementing the ForgeRock Token Vault with the ForgeRock SDK for JavaScript.
First, why advanced token security?
In JavaScript Single Page Applications (or SPA), OAuth/OIDC Tokens (referred to from here on as tokens) are typically stored by using the browser’s Web Storage API: localStorage
or sessionStorage
.
The security mechanism the browser uses to ensure data doesn’t leak out to unintended actors is through the Same-Origin Policy. In short, only JavaScript running on the exact same origin, that is scheme, domain, and port, can access the stored data.
For example, if an SPA running on https://auth.example.com/login
stores data, JavaScript running on the following will be able to access it:
-
https://auth.example.com/users
: origins match, regardless of path -
https://auth.example.com?status=abc
: origins match, regardless of query parameters
The following will NOT be able to access the data:
-
http://auth.example.com
: uses a different scheme,http
vs.https
-
https://auth.examples.com
: uses a different domain; notice the plurality -
https://example.com
: does not include the sub-domain -
https://auth.example.com:8000
: uses a different port
For the majority of web applications, this security model can be sufficient.
In most JavaScript applications, the code running on the app’s origin can usually be trusted; hence, the browser’s Web Storage API is sufficient as long as good security practices are in place.
However, in applications that are high-value targets, such as apps required to run untrusted, third-party code, or apps that have elevated scrutiny due to regulatory or compliance needs, the Same-Origin Policy may not be enough to protect the stored tokens.
Examples of situations where the Same-Origin Policy may not be sufficient include government agencies, financial organizations, or those that store sensitive data, such as medical records. The web applications of these entities may have enough inherent risk to offset the complexity of a more advanced token security implementation.
There are two solutions that can increase token security:
The Backend for Frontend (BFF) pattern
One solution that is quite common is to avoid storing high-value secrets within the browser in the first place. This can be done with a dedicated Backend for Frontend, or BFF. Yeah, it’s a silly initialism.
This is increasingly becoming a common pattern for apps made with common meta-frameworks, such as Next, Nuxt, SvelteKit, and so on. The server component of these frameworks can store the tokens on the front end’s behalf using in-memory stores or just writing the token into an HTTPOnly cookie, and requests that require authorization can be proxied through the accompanying server where the tokens are accessible. Therefore, no token needs to be stored on the front end, eliminating the risk.
You can read more about the arguments in favor of, and against, this design in the Hacker News discussion on the blog post titled "SPAs are Dead".
If, on the other hand, you are not in the position to develop, deploy and maintain a full backend, you have an alternative choice for securing your tokens: Origin Isolation.
Origin Isolation
Origin Isolation is a concept that introduces an alternative to BFF, and provides a more advanced mechanism for token security.
The concept is to store tokens in a different and dedicated origin related to the main application. To do this, two web servers are needed to respond to two different origins: one is your main app, and the second is an iframed app dedicated to managing tokens.
For more information, refer to ForgeRock’s patent: Transparently using origin isolation to protect access tokens.
This particular design means that if your main application gets compromised, the malicious code running in your main app’s origin still has no access to the tokens stored in the alternative origin.
You’ll still need a web server of some kind to serve the files necessary to handle requests to this alternative origin, but being that only static files are served, the options are much simpler and lightweight.
This solution, which is implemented by using the ForgeRock Token Vault is the focus of this tutorial.
What is ForgeRock Token Vault?
ForgeRock Token Vault is a codified implementation of Origin Isolation. For more information, refer to ForgeRock Token Vault in the ForgeRock documentation.
It is a plugin available to customers that use the ForgeRock SDK for JavaScript to enable OAuth 2.0 or OIDC token request and management in their apps. These apps can remain largely unmodified to take advantage of ForgeRock Token Vault.
Even though your main app doesn’t need much modification, additional build and server requirements are necessary, which introduces complexity and added maintenance to your system. We recommend that you only integrate ForgeRock Token Vault into an app if heightened security measures are a requirement of your system. |
What you will learn
We will use an existing React JS, to-do sample application similar to what we built in another guide as a starting point for implementing ForgeRock Token Vault. This represents a realistic web application that has an existing implementation of ForgeRock’s SDK for JavaScript. Unlike the previous tutorial, we’ll start with a fully working app and focus on adding ForgeRock Token Vault.
This tutorial focuses on OAuth 2.0 and OIDC authorization and token management. Authentication-related concerns, such as login and registration journeys are handled outside the app. This is referred to as Centralized login.
This is not a guide on how to build a React app
How you architect or construct React apps is outside the scope of this guide. It’s also worth noting that there are many React-based frameworks and generators for building web applications, such as Next.js, Remix, and Gatsby. What is best is highly contextual to the product and user requirements for any given project. |
To demonstrate ForgeRock Token Vault integration, we will be using a simplified, non-production-ready, to-do app. This to-do app represents a Single Page Application (SPA) with nothing more than React Router added to the stack for routing and redirection.
Using this tutorial
This is a hands-on tutorial. We are providing the web app and resource server for you. You can find the repo on GitHub to follow along.
All you’ll need is your own ForgeRock Identity Cloud or Access Manager. If you don’t have access to either, and you are interested in ForgeRock’s Identity and Access Management platform, reach out to a representative today, and we’ll be happy to get you started.
There are two ways to use this guide:
-
Follow along by building portions of the app yourself: continue by ensuring you can meet the requirements below.
-
Just curious about the code implementation details: skip to Implementing the Token Vault.
Requirements
Knowledge requirements
-
JavaScript and the npm ecosystem of modules
-
The command line interface, such as Shell or Bash
-
Core Git commands, including
clone
andcheckout
-
React and basic React conventions
-
Context API: the concept for managing global state
-
Build systems/bundlers and development servers: We use basic Vite configuration and commands
ForgeRock setup
If you’ve already completed the previous tutorial for React JS or Angular, then you may already have most of this setup within your ForgeRock server. We’ll call out the newly introduced data points to ensure you don’t miss the configuration. |
Step 1. Configure CORS (Cross-Origin Resource Sharing)
Due to the fact that pieces of our system will be running on different origins (scheme, domain, and port), we need to configure CORS in the ForgeRock server to allow our web app to make requests. Use the following values:
-
Allowed Origins:
http://localhost:5173
http://localhost:5175
-
Allowed Methods:
GET
POST
-
Allowed headers:
accept-api-version
authorization
content-type
x-requested-with
-
Allow credentials: enable
Rather than domain aliases and self-signed certificates, we will use localhost
as that is a trusted domain by default. The main application will be on the 5173
port, and the proxy will be on the 5175 port. Because the proxy also make calls to the AM server, its origin must also be allowed.
Service Workers are not compatible with self-signed certificates, regardless of tool or creation method. Certificates from a system-trusted, valid Certificate Authority (CA) are required or direct use of
|
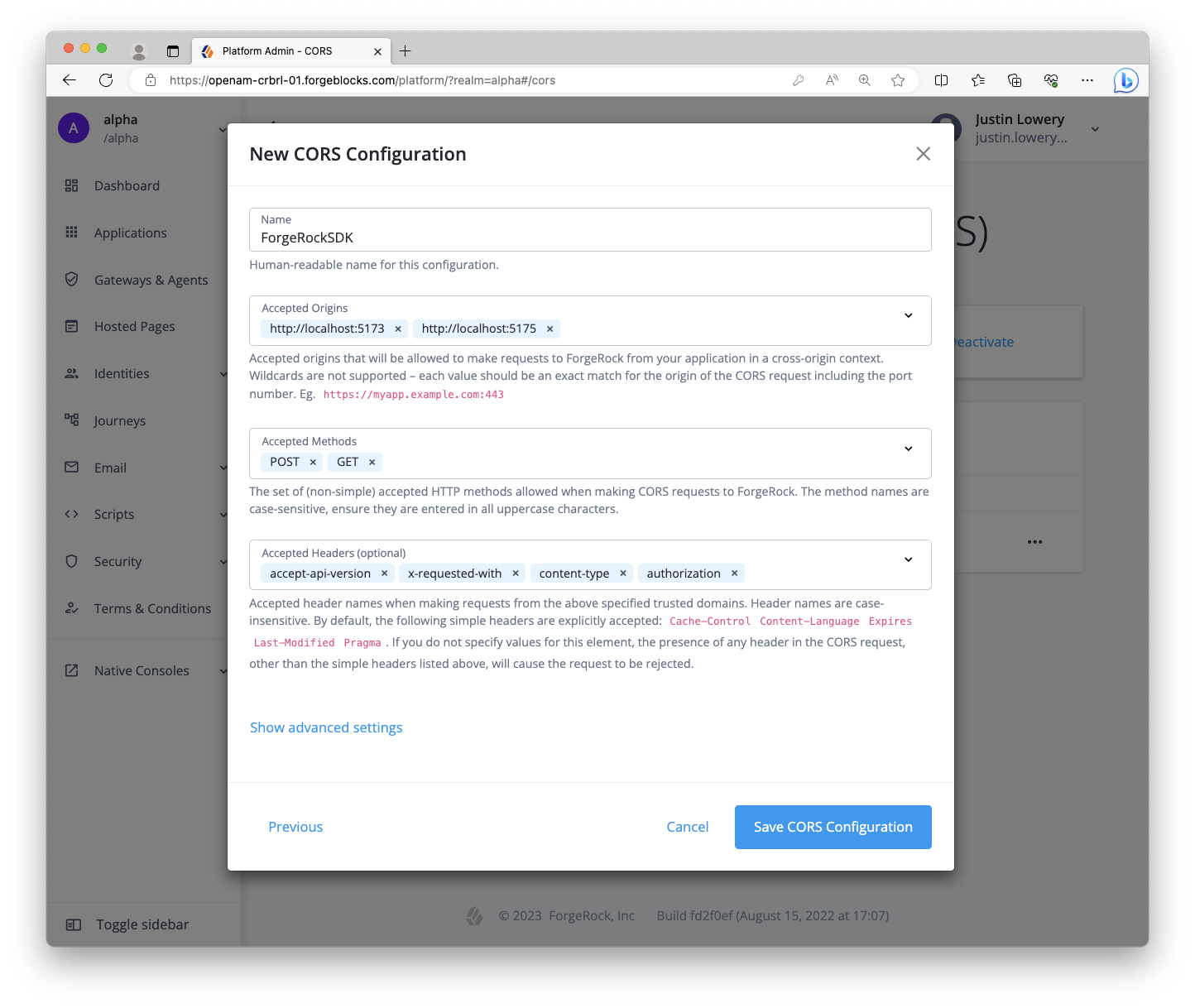
For more information about CORS configuration, refer to the following:
Step 2. Create two OAuth 2.0 clients
Within the ForgeRock server, create two OAuth 2.0 clients: one for the React web app and one for the Node.js resource server.
Why two? It’s conventional to have one OAuth 2.0 client per app in the system. For this case, a public OAuth 2.0 client for the React app provides our app with OAuth 2.0 or OIDC tokens. The Node.js server validates the user’s Access Token shared via the React app using its own confidential OAuth 2.0 client.
Public OAuth 2.0 client settings
-
Client name/ID:
CentralLoginOAuthClient
-
Client type:
Public
-
Secret:
<leave empty>
-
Scopes:
openid
profile
email
-
Grant types:
Authorization Code
Refresh Token
-
Implicit consent: enabled
-
Redirection URLs/Sign-in URLs:
http://localhost:5173/login
-
Response types:
code
id_token
refresh_token
-
Token authentication endpoint method:
none
The client name and redirection/sign-in URL values have changed from the previous tutorial, as well as the Refresh Token grant and response type values. |
Confidential OAuth 2.0 client settings
-
Client name/ID:
RestOAuthClient
-
Client type:
Confidential
-
Secret:
<alphanumeric string>
(treat it like a password) -
Default scope:
am-introspect-all-tokens
-
Grant types:
Authorization Code
-
Token authentication endpoint method:
client_secret_basic
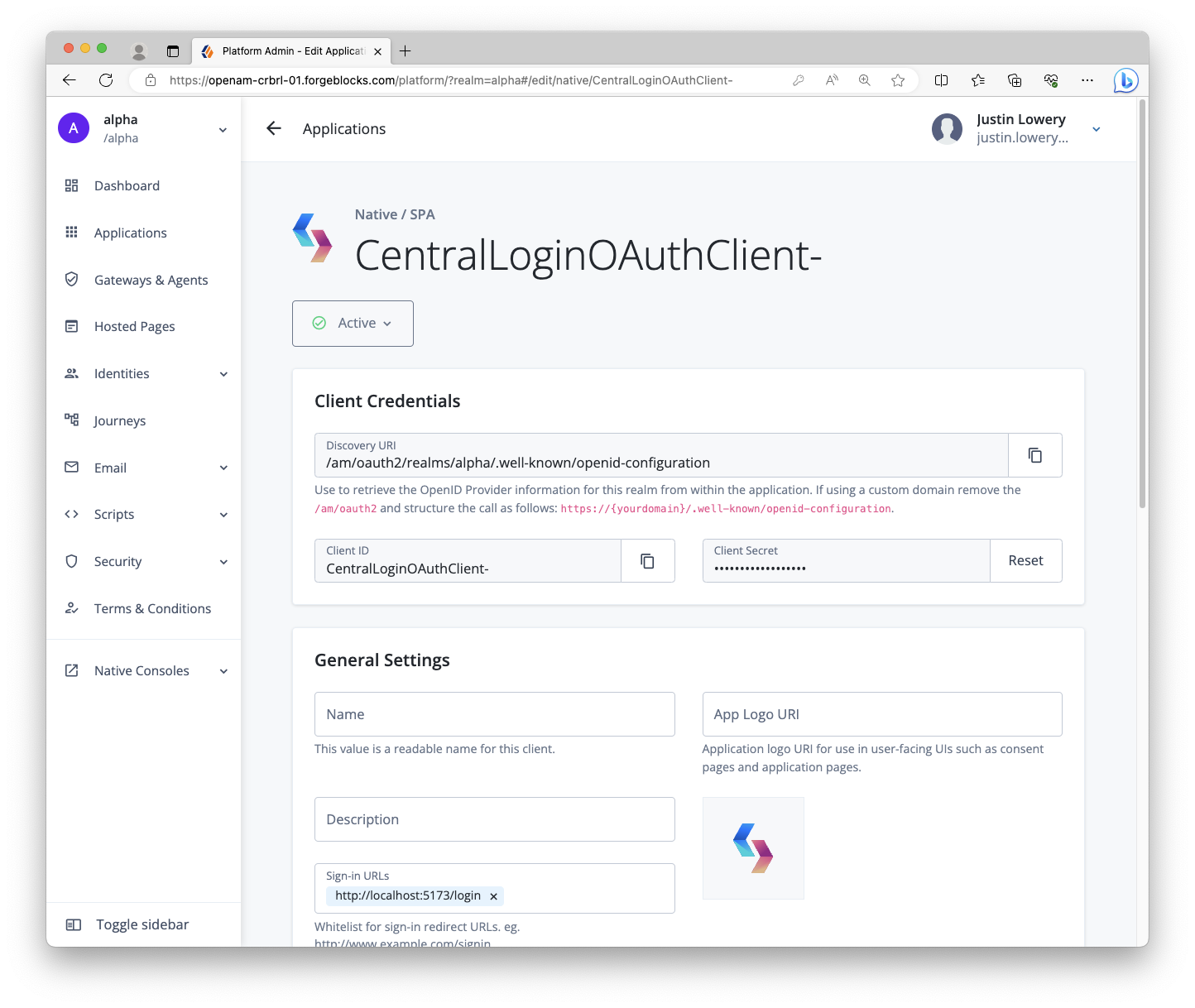
Select the environment you are using for more information on configuring OAuth 2.0 clients:
Local project setup
Step 1. Installing the project
First, clone the JavaScript SDK project to your local computer, cd
(change directory) into the project folder, check out the branch for this guide, and install the needed dependencies:
git clone https://github.com/cerebrl/forgerock-sample-web-react-ts
cd forgerock-sample-web-react-ts
git checkout blog/token-vault-tutorial/start
npm install
There’s also a branch that represents the completion of this guide. If you get stuck, you can check out the blog/token-vault-tutorial/complete branch from GitHub.
|
Step 2. Create an .env
file
First, open the .env.example
file in the root directory. Copy this file and rename it .env
. Add your relevant values to this new file as it provides all the important configuration settings to your applications.
Here’s a hypothetical example .env
file:
.env
file# .env
# System settings
VITE_AM_URL=https://openam-forgerock-sdks.forgeblocks.com/am/ # Needs to be your ForgeRock server
VITE_APP_URL=http://localhost:5173
VITE_API_URL=http://localhost:5174
VITE_PROXY_URL=http://localhost:5175 # This will be our Token Vault Proxy URL
# AM settings
VITE_AM_JOURNEY_LOGIN=Login # Not used with Centralized Login
VITE_AM_JOURNEY_REGISTER=Registration # Not used with Centralized Login
VITE_AM_TIMEOUT=50000
VITE_AM_REALM_PATH=alpha
VITE_AM_WEB_OAUTH_CLIENT=CentralLoginOAuthClient
VITE_AM_WEB_OAUTH_SCOPE=openid email profile
# AM settings for your API (todos) server
# (does not need VITE prefix)
AM_REST_OAUTH_CLIENT=RestOAuthClient
AM_REST_OAUTH_SECRET=6MWE8hs46k68g9s7fHOJd2LEfv # Don't use; this is just an example
We are using Vite for our client apps' build system and development server, so by using the VITE_
prefix, Vite automatically includes these environment variables in our source code. Here are descriptions for some of the values:
-
VITE_AM_URL
: This should be your ForgeRock server and the URL almost always ends with/am/
-
VITE_APP_URL
,VITE_API_URL
, andVITE_PROXY_URL
: These will be the URLs you use for your locally running apps -
VITE_AM_REALM_PATH
: The realm of your ForgeRock server. Likely,alpha
if using Identity Cloud orroot
if using a self-managed AM server -
VITE_REST_OAUTH_CLIENT
andVITE_REST_OAUTH_SECRET
: This is the OAuth 2.0 client you configure in your ForgeRock server to support the REST API server
Build and run the project
Now that everything is set up, build and run the to-do app project. Open two terminal windows and use the following commands in the root directory of the SDK repo:
# Start the React app
npm run dev:react
This dev:react
command uses Vite restarts on any change to a dependent file. This also applies to the dev:proxy
we will build shortly.
# Start the Rest API
npm run dev:api
This dev:api
command runs a basic Node server with no "watchers." This should not be relevant as you won’t have to modify any of its code. If a change is made within the todo-api-server
workspace or an environment variable it relies on, a restart would be required.
We use npm workspaces to manage our multiple sample apps but understanding how it works is not relevant to this tutorial. |
Open the app in browser
In a different browser than the one you are using to administer the ForgeRock server, visit the following URL: http://localhost:5173
. For example, you could use Edge for the app development and Chrome for the ForgeRock server administration.
A home page should be rendered explaining the purpose of the project. It should look like the example below, but it might be a dark variant if you have the dark theme/mode set in your OS:
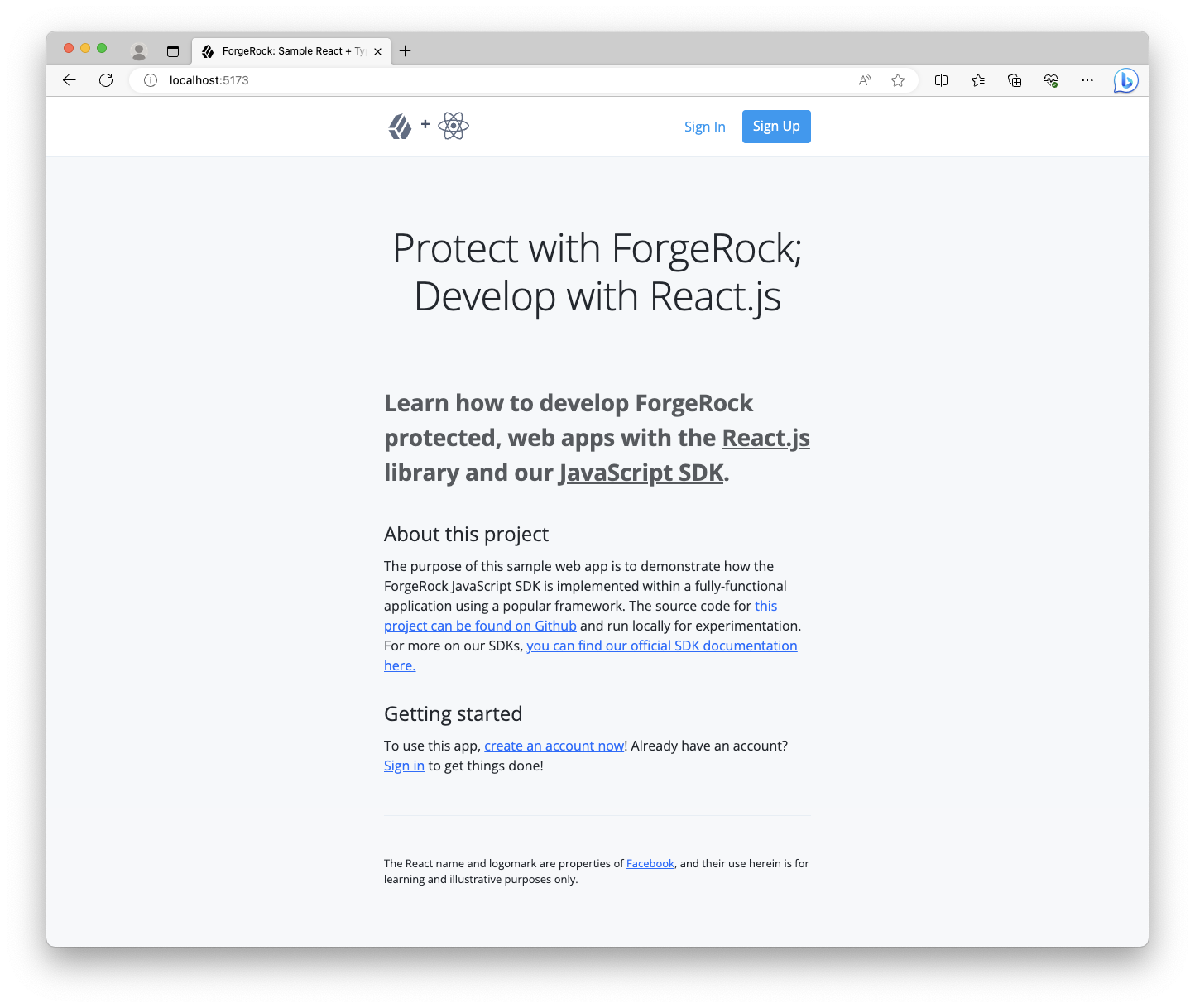
If you encounter errors, here are a few tips:
-
Visit
http://localhost:5174/healthcheck
in the same browser you use for the React app; ensure it responds with "OK" -
Check the terminal that has the
dev:react
command running for error output -
Ensure you are not logged into the ForgeRock server within the same browser as the sample app; log out from ForgeRock if you are and use a different browser
Click the Sign in link in the header or in the Getting started section to sign in to the app with your test user. After successfully authenticating, you should see the app respond to the existence of the valid tokens.
Open your browser’s developer tools to inspect its localStorage
. You should see a single origin with an object containing tokens:
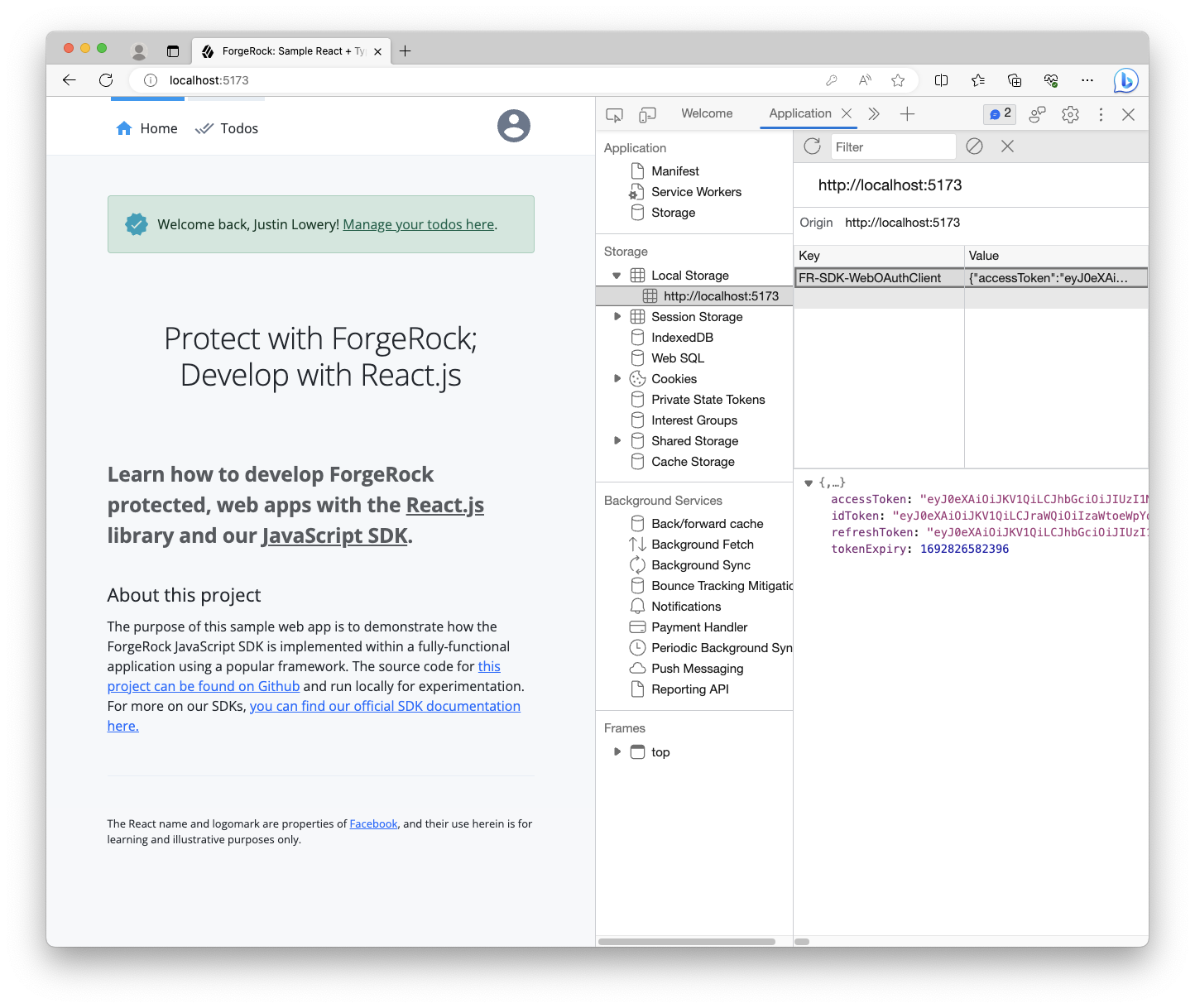
Install Token Vault module
Install the Token Vault npm module within the root of the app:
npm install @forgerock/token-vault
This npm module is used throughout multiple applications in our project, so installing it at the root rather than at the app or workspace level is a benefit.
Implement the Token Vault Proxy
Step 1. Scaffold the Proxy
Next, we’ll need to create a third application, the Token Vault Proxy.
Follow this structure when creating the new directory and its files:
root
├── todo-api-server/
├── todo-react-app/
+ └─┬ token-vault-proxy/
+ ├─┬ src/
+ │ └── index.ts
+ ├── index.html
+ ├── package.json
+ └── vite.config.ts
Step 2. Add the npm workspace
To ease some of the dependency management and script running, add this new "workspace" to our root package.json
, and then add a new script to our scripts
:
package.json
file@@ package.json @@
@@ collapsed @@
"scripts": {
"clean": "git clean -fdX -e \"!.env\"",
"build:api": "npm run build --workspace todo-api-server",
"build:react": "npm run build --workspace todo-react-app",
+ "build:proxy": "npm run build --workspace token-vault-proxy",
"dev:api": "npm run dev --workspace todo-api-server",
"dev:react": "npm run dev --workspace todo-react-app",
+ "dev:proxy": "npm run dev --workspace token-vault-proxy",
"dev:server": "npm run dev --workspace todo-api-server",
"lint": "eslint --ext ts,tsx --report-unused-disable-directives --max-warnings 0",
"serve:api": "npm run serve --workspace todo-api-server",
- "serve:react": "npm run serve --workspace todo-react-app"
+ "serve:react": "npm run serve --workspace todo-react-app",
+ "serve:proxy": "npm run serve --workspace token-vault-proxy"
},
@@ collapsed @@
"workspaces": [
"todo-api-server"
- "todo-react-app"
+ "todo-react-app",
+ "token-vault-proxy",
]
Step 3. Setup the supporting files
Create a new directory at the root named token-vault-proxy
, then create a package.json
file:
package.json
file@@ token-vault-proxy/package.json @@
+ {
+ "name": "token-vault-proxy",
+ "private": true,
+ "version": "1.0.0",
+ "description": "The proxy for Token Vault",
+ "main": "index.js",
+ "scripts": {
+ "dev": "vite",
+ "build": "vite build",
+ "serve": "vite preview --port 5175"
+ },
+ "dependencies": {},
+ "devDependencies": {},
+ "license": "MIT"
+ }
Now, create the Vite config file:
@@ token-vault-proxy/vite.config.ts @@
+ import { defineConfig, loadEnv } from 'vite'; (1)
+
+ // https://vitejs.dev/config/
+ export default defineConfig(({ mode }) => { (2)
+ const env = loadEnv(mode, `${process.cwd()}/../`); (3)
+ const port = Number(new URL(env.VITE_APP_URL).port); (4)
+
+ return { (5)
+ envDir: '../', // Points to the `.env` created in the root dir
+ root: process.cwd(),
+ server: {
+ port,
+ strictPort: true,
+ },
+ };
+ });
What does the above do? Good question! Let’s review it:
1 | We import helper functions from vite |
2 | Using defineConfig , we pass in a function, as opposed to an object, because we want to calculate values at runtime |
3 | The parameter mode helps inform Vite how the config is being executed, useful when you need to calculate env variables |
4 | Then, extract the port out of our app’s configured origin, which should be 5175 |
5 | Finally, use this data to construct the config object and return it |
Now, create the index.html
file. This file can be overly simple as all you need is the inclusion of the JavaScript file that will be our proxy:
index.html
file@@ token-vault-proxy/index.html @@
+ <!DOCTYPE html>
+ <html>
+ <p>Proxy is OK</p>
+ <script type="module" src="src/index.ts"></script>
+ </html>
If you’re not familiar with how Vite works, seeing the .ts
extension may look a bit odd in an HTML file but don’t worry. Vite uses this to find entry files, and it rewrites the actual .js
reference for us.
Step 4. Create and configure the Proxy
Let’s create and configure the Token Vault Proxy according to our needs. First, create the src
directory and the index.ts
file within it.
index.ts
file@@ src/index.ts @@
+ import { proxy } from '@forgerock/token-vault';
+
+ // Initialize the token vault proxy
+ proxy({
+ app: {
+ origin: new URL(import.meta.env.VITE_APP_URL).origin, (1)
+ },
+ forgerock: { (2)
+ clientId: import.meta.env.VITE_AM_WEB_OAUTH_CLIENT,
+ scope: import.meta.env.VITE_AM_WEB_OAUTH_SCOPE,
+ serverConfig: {
+ baseUrl: import.meta.env.VITE_AM_URL,
+ },
+ realmPath: import.meta.env.VITE_AM_REALM_PATH,
+ },
+ proxy: { (3)
+ urls: [`${import.meta.env.VITE_API_URL}/*`],
+ }
+ });
The configuration above represents the minimum needed to create the Token Vault Proxy:
1 | We need to declare the app’s origin, as that’s the only source to which the Proxy will respond. |
2 | We have the ForgeRock configuration in order for the Proxy to call out to the ForgeRock server effectively for token lifecycle management. |
3 | Finally, there’s the Proxy’s urls array that acts as an allow-list to ensure only valid URLs are proxied with the appropriate tokens. |
Step 5. Build and verify the Proxy
With everything set up, build the proxy app and verify it’s being served correctly.
npm run dev:proxy
Once the script finishes its initial build and runs the server, you can now check the app and ensure it’s running. Go to http://localhost:5175
in your browser. You should see "Proxy is OK" printed on the screen, and there should be no errors in the Console or Network tab of your browser’s dev tools.
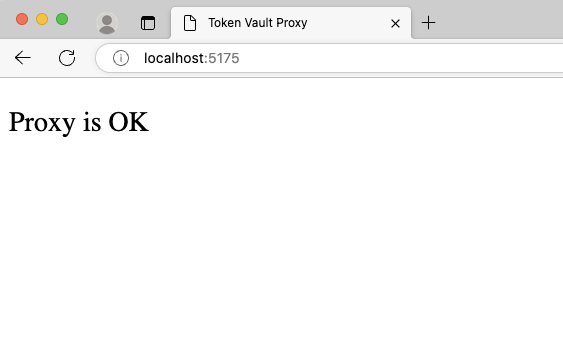
Implement the Token Vault Interceptor
Step 1. Create the Token Vault Interceptor build config
Since the Token Vault Interceptor is a Service Worker, it needs to be bundled separately from your main application code. To do this, write a new Vite config file within the todo-react-app
directory/workspace named vite.interceptor.config.ts
.
We do not recommend trying to use the same configuration file for both your app and Interceptor.
root
├── ...
├─┬ todo-react-app/
│ ├── ...
│ ├── vite.config.ts
+ │ └── vite.interceptor.config.ts
Now that you have the new Vite config for the Interceptor, import the defineConfig
method and pass it the appropriate configuration.
vite.interceptor.config.ts
file@@ todo-react-app/vite.interceptor.config.ts @@
+ import { defineConfig } from 'vite';
+
+ // https://vitejs.dev/config/
+ export default defineConfig({
+ build: {
+ emptyOutDir: false,
+ rollupOptions: {
+ input: 'src/interceptor/index.ts',
+ output: {
+ dir: 'public', // Treating this like a static asset is important
+ entryFileNames: 'interceptor.js',
+ format: 'iife', // Very important for better browser support
+ },
+ },
+ },
+ envDir: '../', // Points to the `.env` created in the root dir
+ });
Rather than passing a function into This is because we don’t need any variables at runtime, like env values. |
In the above, we provide the Token Vault Interceptor source file as the input, and then explicitly tell Vite to bundle it as an IIFE (Immediately Invoked Function Expression) and save the output to this app’s public
directory. This means the Token Vault Interceptor will be available as a static asset at the root of our web server.
It is important to know that bundling it as an IIFE and configuring the output to the public directory is intentional and important. Bundling as an IIFE removes any module system from the file, which is vital to supporting all major browsers within the Service Worker context. Outputting it to the public directory like a static asset is also important. It allows the scope
of the Service Worker to also be available at the root.
For more information, refer to Service Worker scopes on MDN.
Step 2. Create the new Token Vault Interceptor file
Let’s create the new Token Vault Interceptor source file that is expected as the entry file to our new Vite config.
root
├─┬ todo-react-app
│ ├─┬ src/
+ │ │ ├─┬ interceptor/
+ │ │ │ └── index.ts
│ │ └── ...
│ ├── ...
│ └── vite.interceptor.config.ts
Step 3. Import and initialize the interceptor
module
Configure your Token Vault Interceptor with the following variables from your .env
file.
index.ts
source file@@ todo-react-app/src/interceptor/index.ts @@
+ import { interceptor } from "@forgerock/token-vault";
+
+ interceptor({
+ interceptor: {
+ urls: [`${import.meta.env.VITE_API_URL}/*`], (1)
+ },
+ forgerock: { (2)
+ serverConfig: {
+ baseUrl: import.meta.env.VITE_AM_URL,
+ timeout: import.meta.env.VITE_AM_TIMEOUT,
+ },
+ realmPath: import.meta.env.VITE_AM_REALM_PATH,
+ },
+ });
The above only covers the minimal configuration needed, but it’s enough to get a basic Interceptor started.
1 | The urls array represents all the URLs you’d like intercepted and proxied through the Token Vault Proxy in order for the Access Token to be added to the outbound request. This should only be for requesting your "protected resources."
The wildcard ( |
2 | The ForgeRock configuration must match the configuration in the main app. This is easily enforced by using the .env file |
Step 4. Build the Interceptor
Now that we have the dedicated Vite config and the Token Vault Interceptor entry file created, add a dedicated build command to the package.json
within the todo-react-app
workspace.
package.json
file@@ todo-react-app/package.json @@
@@ collapsed @@
"scripts": {
- "dev": "vite",
+ "dev": "npm run build:interceptor && vite",
- "build": "vite build",
+ "build": "npm run build:interceptor && vite build",
+ "build:interceptor": "vite build -c ./vite.interceptor.config.ts",
"serve": "vite preview --port 5173"
},
@@ collapsed @@
It’s worth noting that the Token Vault Interceptor will only be rebuilt at the start of the command and not rebuilt after any change thereafter as there’s no watch
command used here for the Token Vault Interceptor itself. Once this portion of code is correctly set up, it should rarely change, so this should be fine.
Your main app will still be rebuilt and "hot-reloading" will take place.
Enter npm run build:interceptor -w todo-react-app
to run the new command you just wrote above in the todo-react-app
workspace. You can see the resulting interceptor.js
built and placed into your public
directory.
root
├─┬ todo-react-app
│ ├─┬ public/
│ │ ├── ...
+ │ │ ├── interceptor.js
Step 5. Ensure interceptor.js
is accessible
Since we haven’t implemented the Token Vault Interceptor yet in the main app, we can’t really test it; however, we can at least make sure the file is accessible in the browser as we expect. To do this, run the following command:
npm run dev:react
After the command starts the server, using your browser, visit http://localhost:5173/interceptor.js
.
You should plainly see the fully built JavaScript file. Ensure it does not have any import
statements and looks complete. It should contain more code than just the original source file you wrote above.
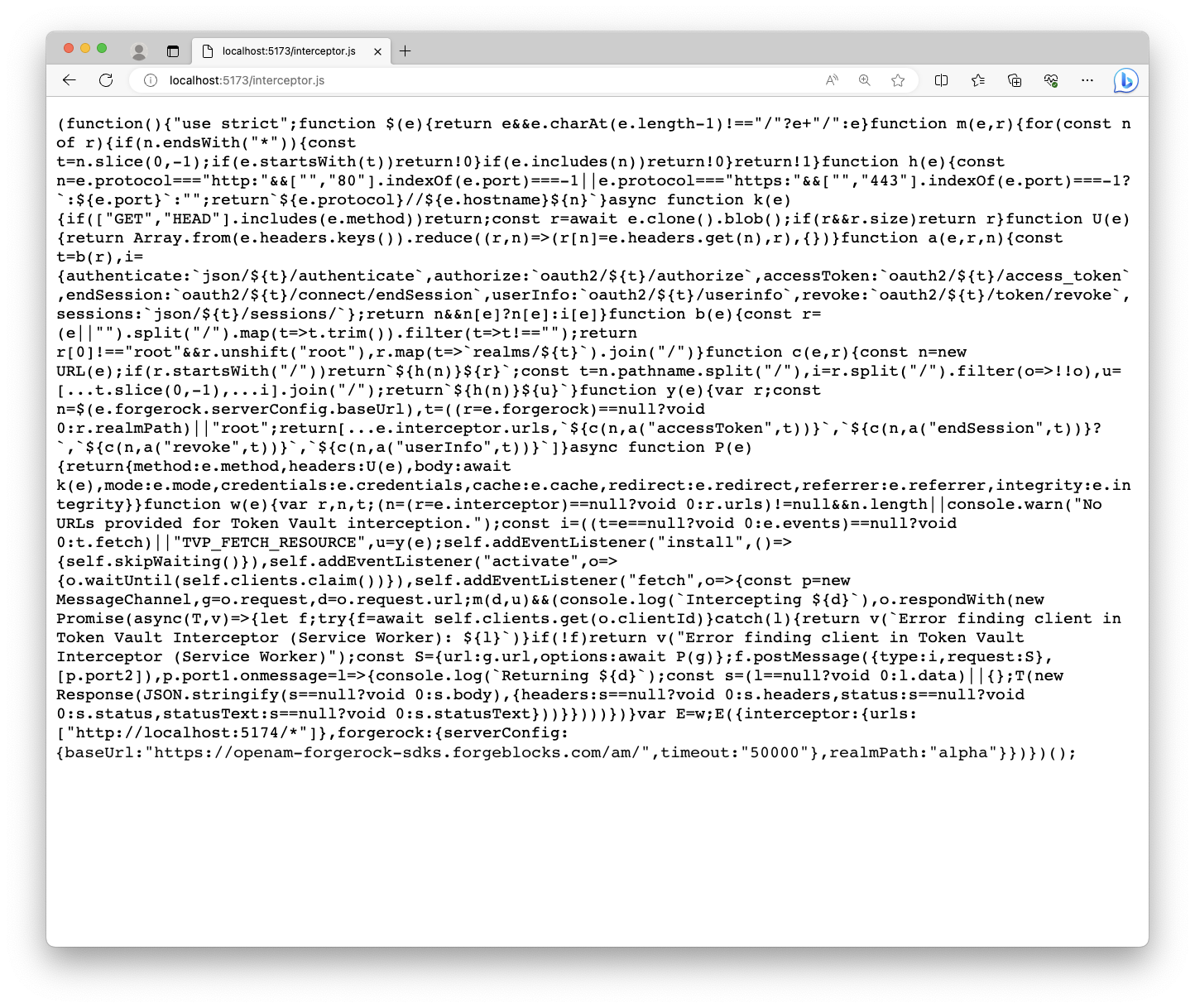
Implement the Token Vault Client
Now that we have all the separate pieces set up, wire it all together with the Token Vault Client plugin.
Step 1. Add HTML element to index.html
When we initiate the Token Vault Proxy, it needs a real DOM element to mount to. The easiest way to ensure we have a proper element is to add it to the index.html
directly.
index.html
file@@ todo-react-app/index.html @@
@@ collapsed @@
<body>
<!-- Root div for mounting React app -->
<div id="root" class="cstm_root"></div>
+
+ <!-- Root div for mounting Token Vault Proxy (iframe) -->
+ <div id="token-vault"></div>
<!-- Import React app -->
<script type="module" src="/src/index.tsx"></script>
</body>
</html>
Step 2. Import and initialize the client
module
First, import the client
module and remove the TokenStorage
module from the SDK import.
Second, call the client
function with the below minimal configuration. This is how we "glue" the three entities together within your main app. This function returns an object that we use to register and instantiate each entity.
index.tsx
source file@@ todo-react-app/src/index.tsx @@
- import { Config, TokenStorage } from '@forgerock/javascript-sdk';
+ import { Config } from '@forgerock/javascript-sdk';
+ import { client } from '@forgerock/token-vault';
import ReactDOM from 'react-dom/client';
@@ collapsed @@
+ const register = client({
+ app: {
+ origin: c.TOKEN_VAULT_APP_ORIGIN,
+ },
+ interceptor: {
+ file: '/interceptor.js', // references public/interceptor.js
+ },
+ proxy: {
+ origin: c.TOKEN_VAULT_PROXY_ORIGIN,
+ },
+ });
/**
* Initialize the React application
*/
(async function initAndHydrate() {
@@ collapsed @@
Remember, the file
reference within the interceptor
object needs to point to the built Token Vault Interceptor file, which will be located in the public
directory as a static file but served from the root, not the source file itself.
This function ensures the app, Token Vault Interceptor and Token Vault Proxy are appropriately configured.
Step 2. Register the interceptor, proxy, and token store
Now that we’ve initialized and configured the client, we now register the Token Vault Interceptor, the Token Vault Proxy, and the token vault store just under the newly added code from above:
index.tsx
source file@@ todo-react-app/src/index.tsx @@
@@ collapsed @@
proxy: {
origin: c.TOKEN_VAULT_PROXY_ORIGIN,
},
});
+
+ // Register the Token Vault Interceptor
+ await register.interceptor();
+
+ // Register the Token Vault Proxy
+ await register.proxy(
+ // This must be a live DOM element; it cannot be a Virtual DOM element
+ // `token-vault` is the element added in Step 1 above to `todo-react-app/index.html`
+ document.getElementById('token-vault') as HTMLElement
+ );
+
+ // Register the Token Vault Store
+ const tokenStore = register.store();
/**
* Initialize the React application
*/
(async function initAndHydrate() {
@@ collapsed @@
Registering the Token Vault Interceptor is what requests and registers the Service Worker. Calling register.interceptor
returns the ServiceWorkerRegistration
object that can be used to unregister the Service Worker, as well as other functions, if that’s needed. We won’t be implementing that in this tutorial.
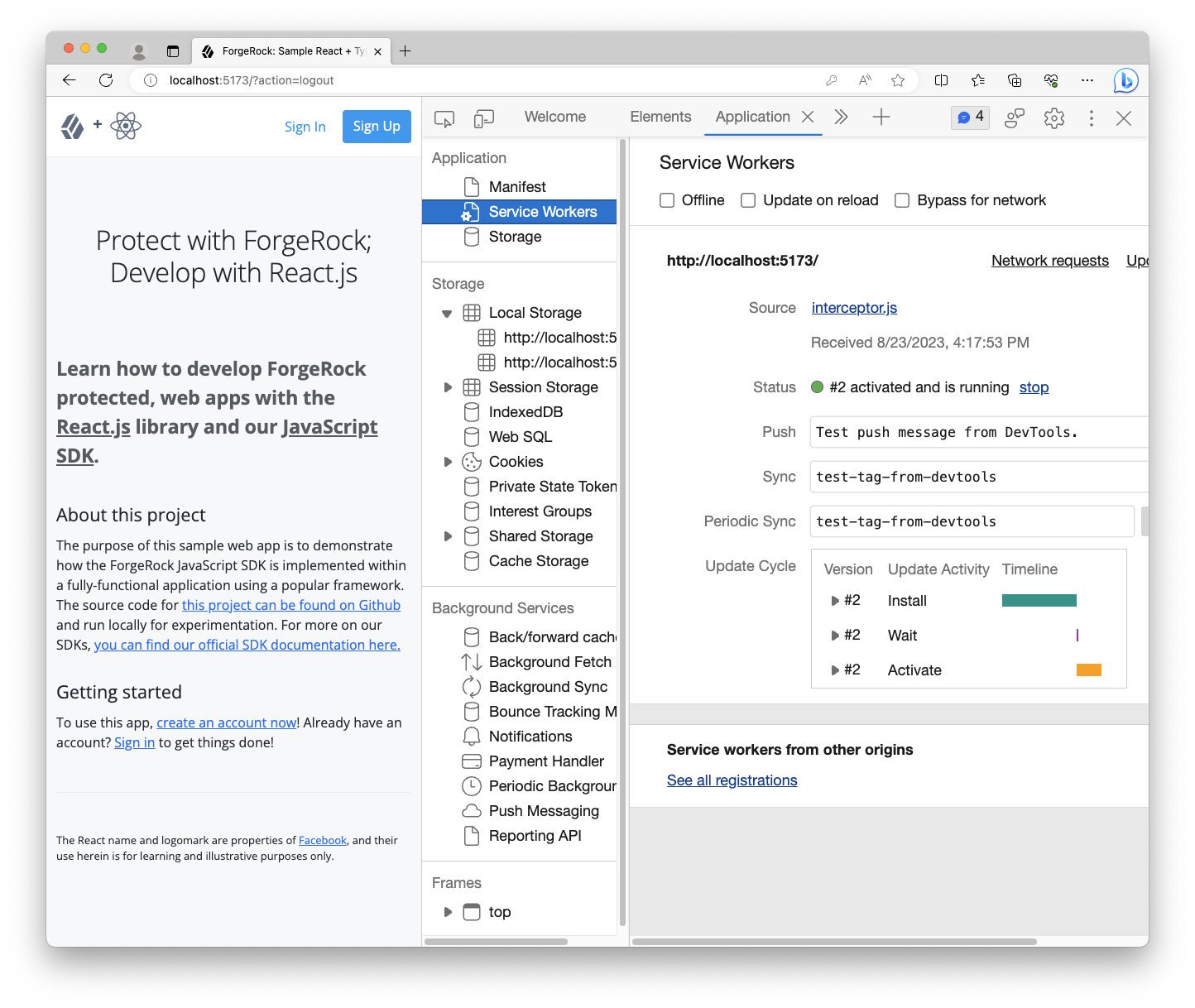
Registering the Token Vault Proxy constructs the iframe
component and mounts it do the DOM element passed into the method. It’s important to note that this must be a real, available DOM element, not a Virtual DOM element. This results in the Token Vault Proxy being "registered" as a child frame and, therefore, accessible to your main app.
Calling register.proxy
also returns an optional reference to the DOM element of the iframe
that can be used to manually destroy the element and the Token Vault Proxy, if needed.
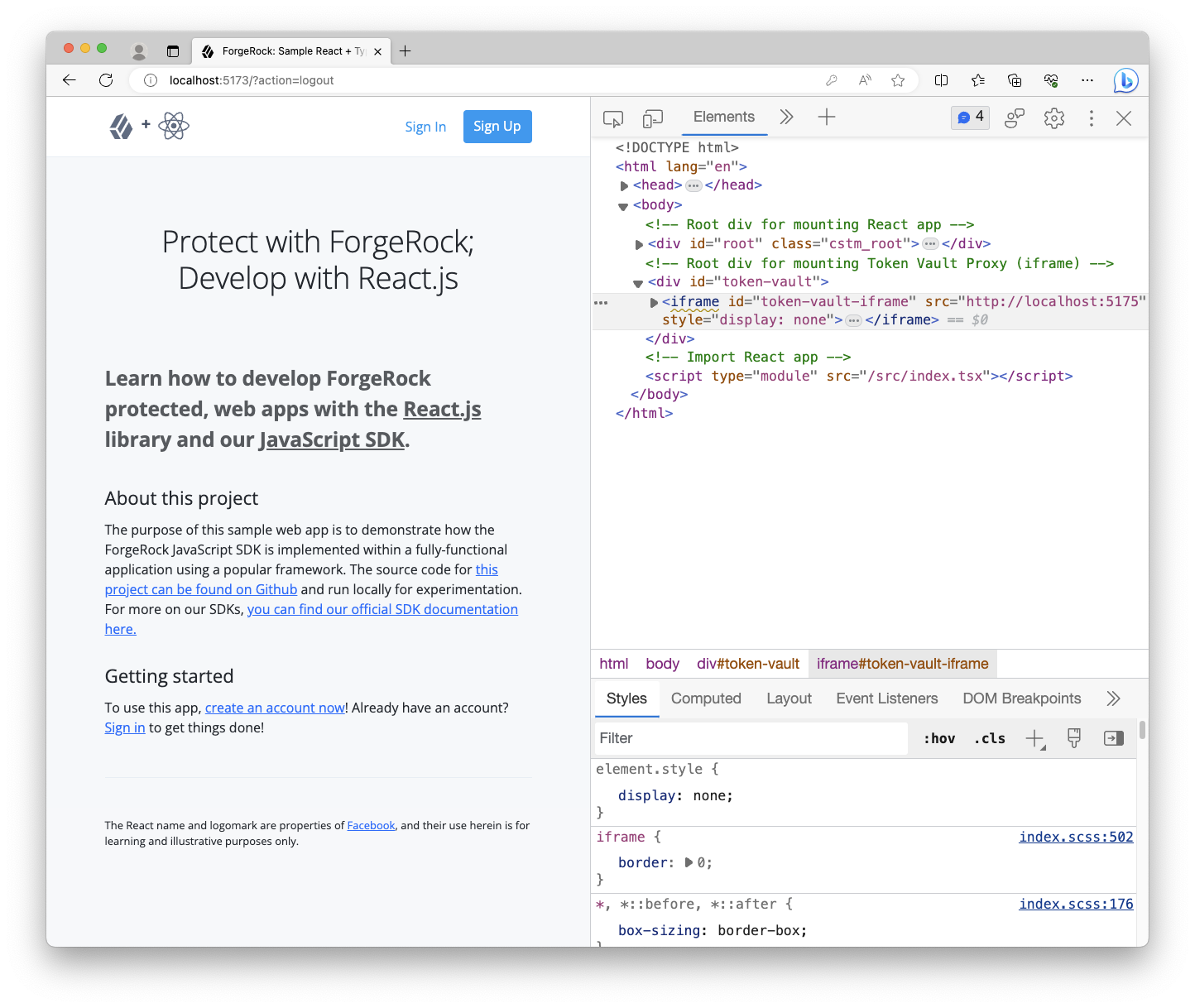
Finally, registering the store provides us with the object that replaces the default token store within the SDK. There are some additional convenience methods on this store
object that we’ll take advantage of later in the tutorial.
You will see a few errors in the console, but don’t worry about those at the moment. The next steps will resolve them.
Step 3. Replace the SDK’s default token store
Within the existing SDK configuration, pass the tokenStore
object we created in the previous step to the set
method to override the SDK’s internal token store.
index.tsx
source file@@ todo-react-app/src/index.tsx @@
@@ collapsed @@
// Configure the SDK
Config.set({
clientId: import.meta.env.WEB_OAUTH_CLIENT,
redirectUri: import.meta.env.REDIRECT_URI,
scope: import.meta.env.OAUTH_SCOPE,
serverConfig: {
baseUrl: import.meta.env.AM_URL,
timeout: import.meta.env.TIMEOUT,
},
realmPath: import.meta.env.REALM_PATH,
+ tokenStore, // this references the Token Vault Store we created above
});
@@ collapsed @@
This configures the SDK to use the Token Vault Store, which is within the Token Vault Proxy, and that it needs to manage the tokens internally.
Step 4. Check for existing tokens
Currently in our application, we check for the existence of stored tokens to provide a hint if our user is authorized. Now that the main app doesn’t have access to the tokens, we have to ask the Token Vault Proxy if it has tokens.
To do this, replace the SDK method of TokenStorage.get
with the Token Vault Proxy has
method:
index.tsx
source file@@ todo-react-app/src/index.tsx @@
@@ collapsed @@
let isAuthenticated = false;
try {
- isAuthenticated = !((await TokenStorage.get()) == null);
+ isAuthenticated = !!(await tokenStore?.has())?.hasTokens;
} catch (err) {
console.error(`Error: token retrieval for hydration; ${err}`);
}
@@ collapsed @@
Note that this doesn’t return the tokens as that would violate the security of keeping them in another origin, but the Token Vault Proxy will inform you of their existence. This is enough to hint to our UI that the user is likely authorized.
Build and run the apps
At this point, all the necessary entities are set up. We can now run all the needed servers and test out our new application with ForgeRock Token Vault enabled.
Open three different terminal windows, all from within the root of this project. Enter each command in its own window:
npm run dev:react
npm run dev:api
npm run dev:proxy
Allow all the commands to complete the build and start the development servers.
Then, visit http://localhost:5173
in your browser of choice. The to-do application should look and behave no different from before.
Open the dev tools of your browser, and proceed to sign in to the app. You will be redirected to the ForgeRock login page, and then redirected back after successfully authenticating. You may notice some additional redirection within the React app itself, this is normal.
Once you land on the home page, you should see the "logged in experience" with your username in the success alert.
To test whether ForgeRock Token Vault is successfully implemented, go to the Application or Storage tab of your dev tools and inspect the localStorage
section.
You should see two origins: http://localhost:5173
, our main app, and http://localhost:5175
, our Token Vault Proxy.
Your user’s tokens should be stored under the Token Vault Proxy origin on port 5175
, not under the React app’s origin on port 5173
.
If you observe that behavior, then you have successfully implemented ForgeRock Token Vault. Congratulations, your tokens are now more securely stored!
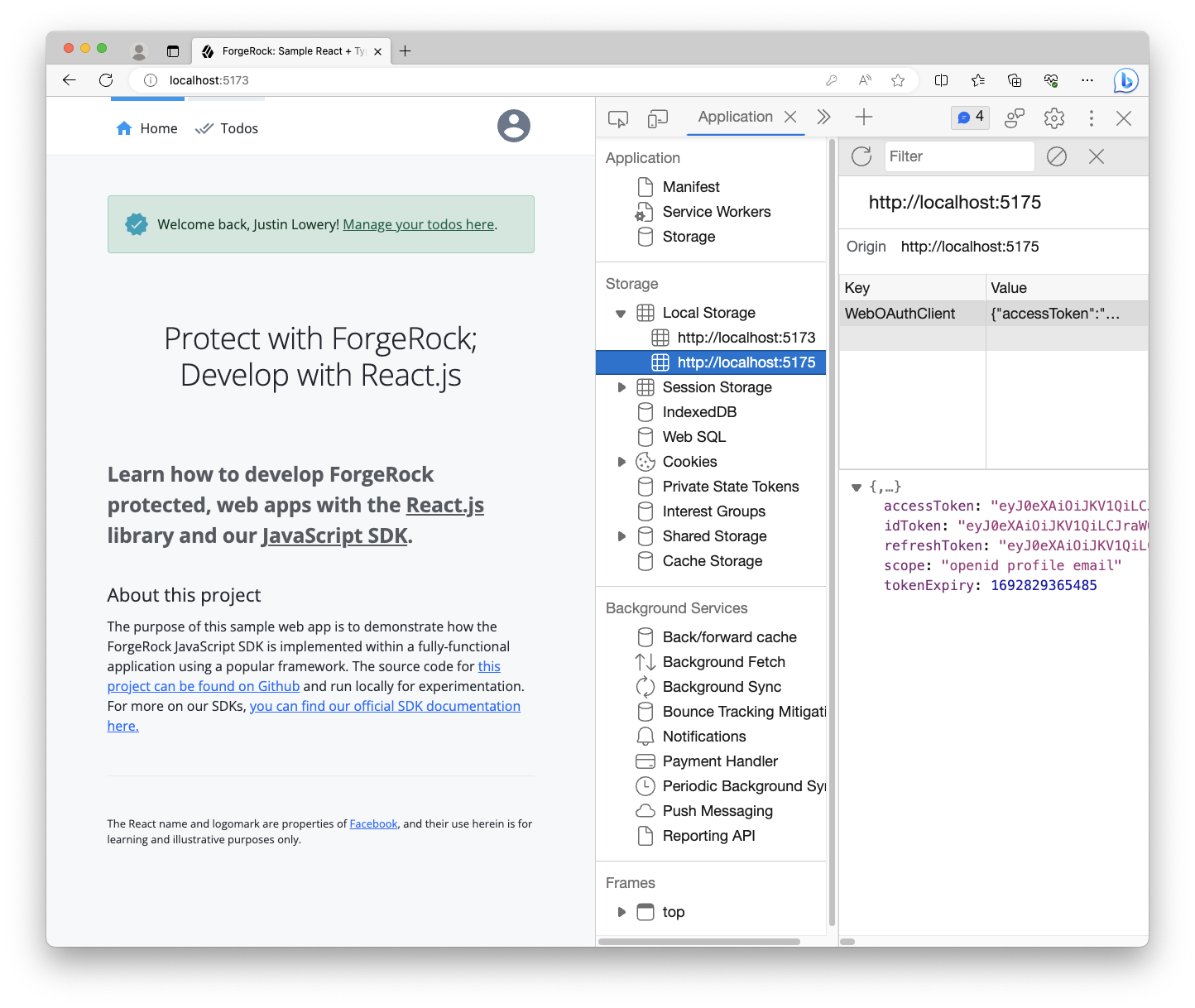
If you don’t see the tokens in the Token Vault Proxy origin’s localStorage
, then follow the troubleshooting section below:
Troubleshooting
Getting failures in the service worker registration
Make sure your dedicated Vite configuration is correct.
Also, check the actual file output for the interceptor.js
file. If the built file has ES Module syntax in it, or it looks incomplete, then it can cause this issue — Service Workers in some browsers, even the latest versions, don’t support the same ES version or features as the main browser context.
Tokens are not being saved under any origin
Open up your browser’s dev tools and ensure the following:
-
Your Token Vault Interceptor is running under your main app’s origin.
-
Do you have an
/access_token
request? It comes after the/authorize
request and redirect. -
Your Token Vault Interceptor is intercepting the
/access_token
request. If it is, you should see two outgoing requests: one for the main app and one for the Token Vault Proxy. -
Your Token Vault Proxy is running within the
iframe
and forwarding the request. -
There are no network errors.
-
There are no console errors.
Enabling Preserve Log for both the Console and the Network tabs is very helpful. |
I’m getting a CORS failure
Make sure you have both origins listed in your ForgeRock CORS configuration.
Additionally, it’s best if you use the ForgeRock SDK template when creating a new CORS config in your ForgeRock server.
If both origins are listed, make sure you have no typos in the allowed methods. The methods like GET
and POST
are case-sensitive. Also, check the headers, which are NOT case-sensitive.
Allow Credentials must be enabled.