Manage organizations over REST
IDM provides RESTful access to managed organizations, at the context path /openidm/managed/realm-name_organization
.
You can add, change, and delete organizations by using the IDM admin UI or over the REST interface.
To use the IDM admin UI, select Manage > Organization.
The examples on this page show how to add, query, modify, and delete organizations over the REST interface. For a reference of all managed organization endpoints and actions, refer to Managed organizations.
Add and modify organizations
The examples in this section create the example-org
organization and add admins and members to the organization.
In this organization, users bjensen and scarter can create and delete child organizations, also known as suborganizations, of example-org
, and can create and delete members within the child organizations:
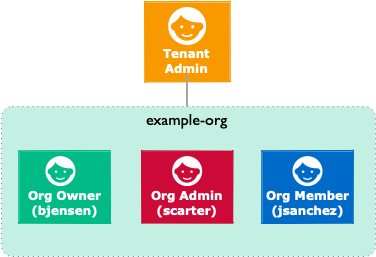
Add a top level organization to IDM.
Only IDM tenant administrators can create top level organizations.
curl \ --header "Authorization: Bearer <token>" \ --header "Content-Type: application/json" \ --header "Accept-API-Version: resource=1.0" \ --header "If-None-Match: *" \ --request PUT \ --data '{ "name": "example-org" }' \ "https://<tenant-env-fqdn>/openidm/managed/realm-name_organization/example-org" { "_id": "example-org", "name": "example-org" ... }
Make a user the organization’s owner.
IDM tenant administrators can designate organizations' owners.
In this example, the Identity Management tenant administrator makes bjensen the owner of the example-org
organization created previously.
The example assumes the user bjensen already exists:
curl \ --header "Authorization: Bearer <token>" \ --header "Content-Type: application/json" \ --header "Accept-API-Version: resource=1.0" \ --request POST \ --data '{ "_ref":"managed/realm-name_user/<bjensenUUID>" }' \ "https://<tenant-env-fqdn>/openidm/managed/realm-name_organization/example-org/owners?_action=create"
Add a member to the organization.
Organization owners can create members in the organizations that they own.
In this example, bjensen creates user scarter.
bjensen also makes scarter a member of the example-org
organization:
curl \ --header "Authorization: Bearer <token>" \ --header "Content-Type: application/json" \ --header "Accept-API-Version: resource=1.0" \ --request POST \ --data '{ "userName": "scarter", "sn": "Carter", "givenName": "Steven", "mail": "scarter@example.com", "password": "Th3Password!", "memberOfOrg": [{"_ref": "managed/realm-name_organization/example-org"}] }' \ "https://<tenant-env-fqdn>/openidm/managed/realm-name_user?_action=create" { ... "mail":"scarter@example.com", "memberOfOrgIDs":["example-org"], ... }
Make one of the organization’s members an admin.
Organization owners can designate admins for the organizations they own.
An organization admin must be a member of the organization.
In this example, bjensen makes scarter an admin of the example-org
organization:
curl \ --header "Authorization: Bearer <token>" \ --header 'Content-Type: application/json' \ --header "Accept-API-Version: resource=1.0" \ --request PATCH \ --data '[ { "operation": "add", "field": "/admins/-", "value": { "_ref": "managed/realm-name_user/<scarterUUID>" } } ]' \ "https://<tenant-env-fqdn>/openidm/managed/realm-name_organization/example-org" { "_id": "example-org", ... "name": "example-org" }
Add a member to the organization.
Organization admins can add members to the organizations they administer.
In this example, the organization admin, scarter, creates a new member, jsanchez.
scarter also makes jsanchez a member of the example-org
organization:
curl \ --header "Authorization: Bearer <token>" \ --header "Content-Type: application/json" \ --header "Accept-API-Version: resource=1.0" \ --request POST \ --data '{ "userName": "jsanchez", "sn": "Sanchez", "givenName": "Juanita", "mail": "jsanchez@example.com", "password": "Th3Password!", "memberOfOrg": [{"_ref": "managed/realm-name_organization/example-org"}] }' \ "https://<tenant-env-fqdn>/openidm/managed/realm-name_user?_action=create" { ... "mail":"jsanchez@example.com", "memberOfOrgIDs":["example-org"], ... }
Create a child organization.
Organization owners and admins can create and manage child organizations of the organizations they own or administer.
In this example, the organization owner, bjensen, creates a new organization named example-child-org
, and makes it a child organization of the example-org
organization:
curl \ --header "Authorization: Bearer <token>" \ --header "Content-Type: application/json" \ --header "Accept-API-Version: resource=1.0" \ --header "If-None-Match: *" \ --request POST \ --data '{ "name": "example-child-org", "parent": {"_ref": "managed/realm-name_organization/example-org"} }' \ "https://<tenant-env-fqdn>/openidm/managed/realm-name_organization?_action=create" { ... "parentIDs":["example-org"], ... "name":"example-child-org" }
When creating a child organization, you need to add custom attributes to the access flags for the For more information on adding and updating privileges that apply to managed organizations, refer to Server configuration. |
Query organizations
The examples in this section demonstrate several ways you can query organizations and organization membership:
List all the organizations a user owns.
This example lists the organizations bjensen owns:
curl \ --header "Authorization: Bearer <token>" \ --header "Accept-API-Version: resource=1.0" \ --request GET \ "https://<tenant-env-fqdn>/openidm/managed/realm-name_user/<bjensenUUID>/ownerOfOrg?_queryFilter=true" { "result": [ { ... "_ref": "managed/realm-name_organization/example-org", "_refResourceCollection": "managed/realm-name_organization", "_refResourceId": "example-org", ... } ], ... }
List an organization’s members.
Organization owners can list the members of the organizations they own.
In this example, bjensen lists the members of the example-org
organization:
curl \ --header "Authorization: Bearer <token>" \ --header "Accept-API-Version: resource=1.0" \ --request GET \ "https://<tenant-env-fqdn>/openidm/managed/realm-name_organization/example-org/members?_queryFilter=true" { "result": [ ... ], "resultCount":1, ... }
List organizations that you own or administer, and list their child organizations.
An organization owner or admin can list the organizations that they own or administer. When a user runs the curl command in this example, it lists:
-
The organizations that the user owns or administers
-
Those organizations' child organizations
curl \ --header "Authorization: Bearer <token>" \ --header "Accept-API-Version: resource=1.0" \ --request GET \ "https://<tenant-env-fqdn>/openidm/managed/realm-name_organization?_queryFilter=true" { "result": [ { "_id":"example-org", "parentIDs":[], "name":"example-org" }, { "_id":"<example-child-orgUUID>", "parentIDs":["example-org"], "name":"example-child-org" } "resultCount":2, ... }
List organizations of which another user is a member.
Organization owners and admins can list all the organizations they own or administer that contain a given user. In this example, scarter lists the organizations of which jsanchez is a member:
curl \ --header "Authorization: Bearer <token>" \ --header "Accept-API-Version: resource=1.0" \ --request GET \ "https://<tenant-env-fqdn>/openidm/managed/realm-name_user/<jsanchezUUID>?_fields=memberOfOrg" { "_id": ... "memberOfOrg":[ { "_ref":"managed/realm-name_organization/example-org", "_refResourceCollection":"managed/realm-name_organization", "_refResourceId":"example-org", ... }